Apple’s engineers could have lengthy acknowledged the widespread need amongst iOS builders to recreate the elegant hero animation featured within the App Retailer app. Understanding the complexity and time funding sometimes required to implement such animations from scratch, Apple has included this characteristic into the iOS 18 SDK.
With this replace, now you can obtain an identical animated transition impact in your personal apps utilizing only a few strains of code. This important enhancement empowers builders to create visually interesting and seamless transitions, elevating the general consumer expertise of their apps.
On this tutorial, we’ll discover how one can leverage the brand new NavigationTransition
protocol and the matchedTransitionSource
modifier to create hero animations throughout view transitions.
The Easy Demo App
Let’s dive right into a demo app to discover the brand new APIs. We’ll start with a easy app that exhibits an inventory of cafes in a regular scroll view. Our objective is to implement a characteristic the place tapping on a restaurant takes you to a brand new display screen displaying a full picture with a hero animation.
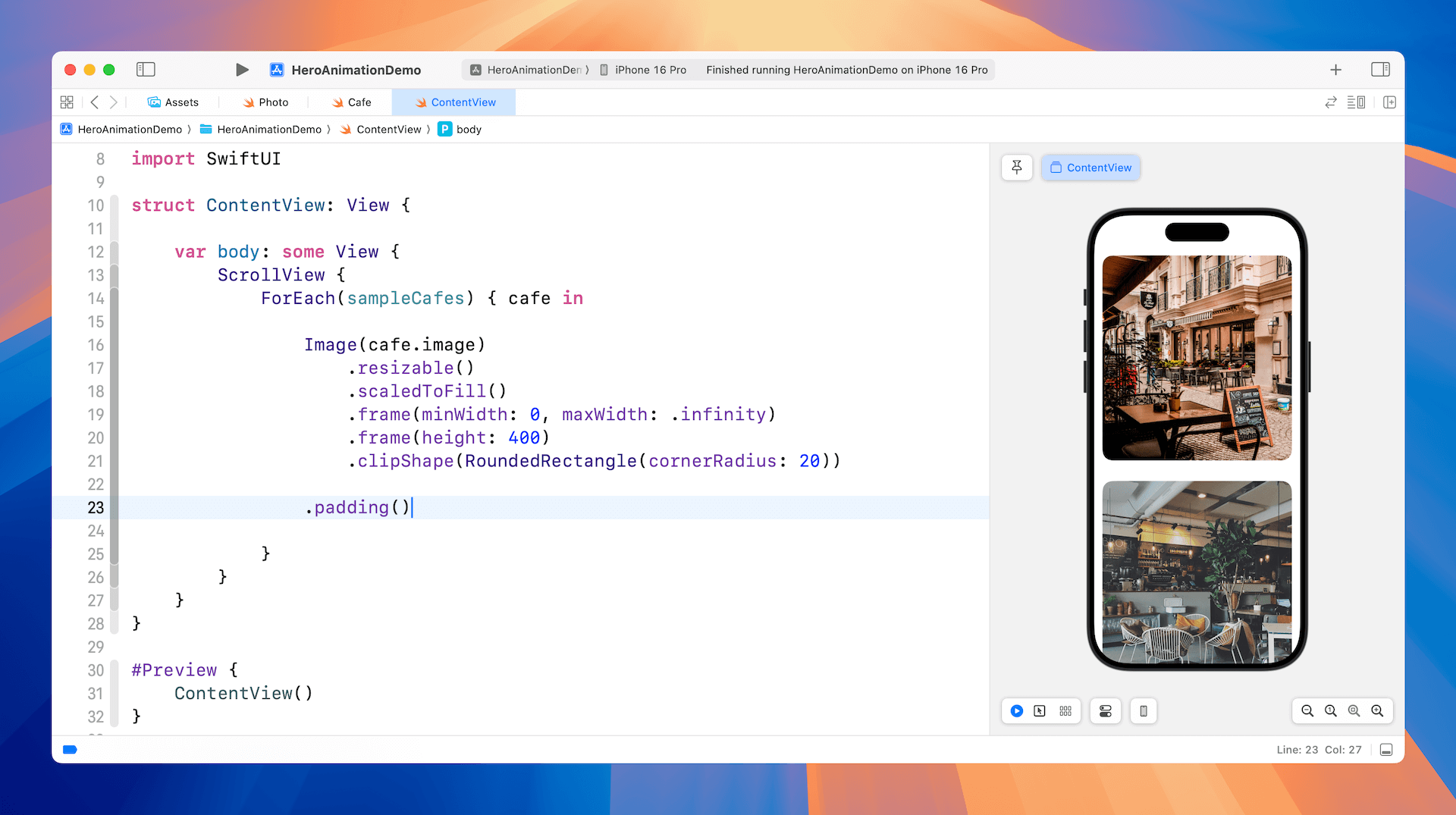
Utilizing Navigation Transition Protocol
To show a full picture and animate the view transition utilizing hero animations, you need to use the next steps:
- Embed the scroll view inside a navigation stack.
- Use
NavigationLink
to allow tapping on the cardboard view. - Declare a namespace with
@Namespace
to help the hero animation. - Connect the
matchedTransitionSource
modifier to the excerpt mode card view. - Connect the
navigationTransition
modifier to the total content material mode card view.
By finishing these steps, SwiftUI will routinely generate a clean hero animation, increasing the chosen cafe merchandise right into a full-screen picture when tapped.
Creating Hero Animations for View Transitions
Now we’ll modify the undertaking to help navigation. To begin, let’s embed the scroll view inside a navigation stack, as proven under:
NavigationStack {
ScrollView {
// Current code
}
}
Subsequent, create the element view for displaying the total picture like under. It accepts a restaurant object as enter and shows its picture in a full-screen view.
struct DetailView: View {
var cafe: Cafe
@Setting(.dismiss) var dismiss
var physique: some View {
Picture(cafe.picture)
.resizable()
.scaledToFill()
.body(minWidth: 0, maxWidth: .infinity)
.clipped()
.overlay(alignment: .topTrailing) {
Button {
dismiss()
} label: {
Picture(systemName: "xmark.circle.fill")
.font(.system(dimension: 30))
.foregroundStyle(Shade.white)
.opacity(0.7)
.padding()
.padding(.high, 30)
}
}
.ignoresSafeArea()
}
}
To allow interplay with the cafe images, we are able to use NavigationLink to handle the navigation. When tapped, the app shows the element view which exhibits the picture in full display screen view.
ForEach(sampleCafes) { cafe in
NavigationLink {
DetailView(cafe: cafe)
} label: {
Picture(cafe.picture)
.resizable()
.scaledToFill()
.body(minWidth: 0, maxWidth: .infinity)
.body(top: 400)
.clipShape(RoundedRectangle(cornerRadius: 20))
}
.padding()
}
In preview mode, you possibly can navigate between the element view and the record view. At this stage, the transition makes use of the default animation for navigation stacks, with none customized results.
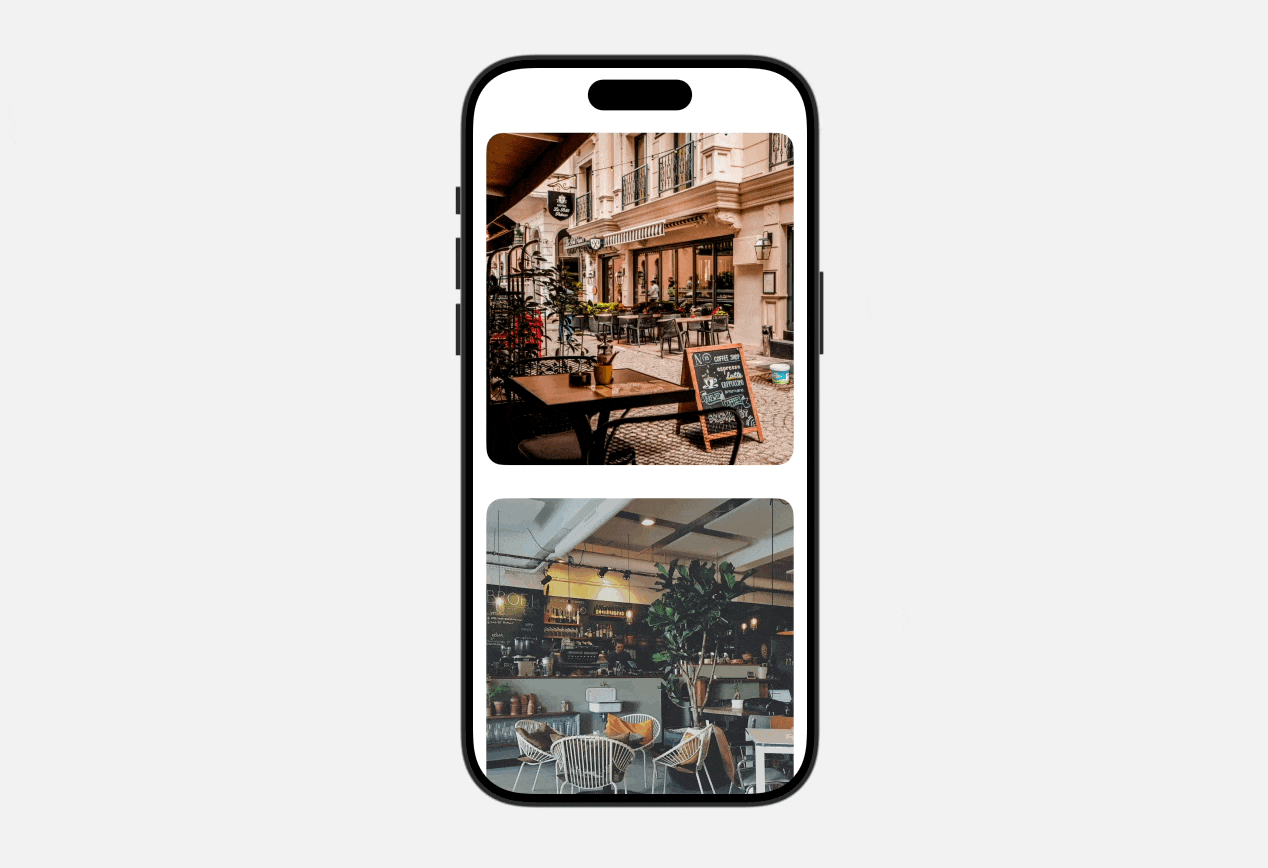
Now it involves the enjoyable half. Let’s create the hero animation by utilizing the brand new NavigationTransition protocol. The very first step is to outline a namespace for the animation. In ContentView
, declare the next namespace variable:
@Namespace var namespace
Subsequent, apply the matchedTransitionSource modifier to the supply view, which is the picture view within the record. Then, use the navigationTransition modifier on the element view. Replace your code as proven under:
NavigationLink {
DetailView(cafe: cafe)
.navigationTransition(.zoom(sourceID: cafe.id, in: namespace))
.toolbarVisibility(.hidden, for: .navigationBar)
} label: {
Picture(cafe.picture)
.resizable()
.scaledToFill()
.body(minWidth: 0, maxWidth: .infinity)
.body(top: 400)
.clipShape(RoundedRectangle(cornerRadius: 20))
.matchedTransitionSource(id: cafe.id, in: namespace)
}
To boost the visible expertise, I’ve included the toolbarVisibility
modifier to hide the navigation bar. This removes the Again button from view, making a extra immersive full-screen presentation of the cafe picture.
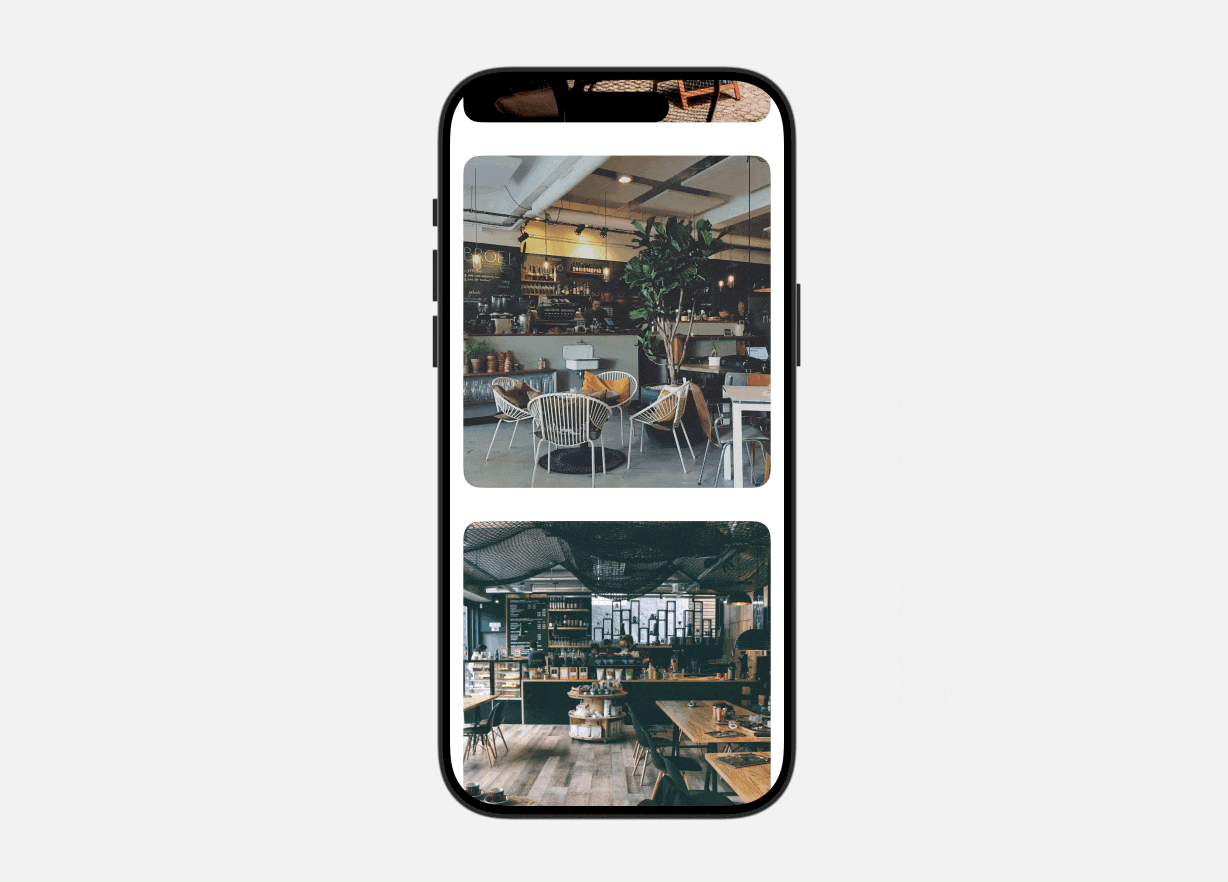
In preview mode, take a look at the app by tapping a restaurant picture. It’ll show a full-screen picture with a hero animation. To return to the record, both faucet the “X” button or just drag the picture downwards. The app will animate the picture again to its authentic place within the record, offering a fluid and intuitive consumer expertise.
Abstract
The brand new NavigationTransition
protocol has made it remarkably easy for builders to create hero animations for view transitions, permitting for a richer consumer expertise with only a few strains of code. Think about exploring this new characteristic to raise your app’s interactivity and consumer satisfaction.
It is vital to notice that this API is just suitable with iOS 18 and later. In case your app must help older iOS variations, you may need to implement the animation your self. Our “Mastering SwiftUI” e-book supplies steering on how one can obtain this.