Companies at this time deal with a big quantity of queries from prospects, gross sales groups, and inner stakeholders. Manually responding to those queries is a sluggish and inefficient course of, usually resulting in delays and inconsistent solutions. A question decision system powered by AI ensures quick, correct, and scalable responses. It really works by retrieving related info and producing exact solutions utilizing Retrieval-Augmented Era (RAG). On this article, I can be sharing with you my journey of constructing a RAG-based question decision system utilizing LangChain, ChromaDB, and CrewAI.
Why Do We Want an AI-powered Question Decision System?
Now, handbook responses take time and should, subsequently, result in delays. Prospects anticipate on the spot replies, and companies want fast entry to correct info. An AI-driven system automates question dealing with, lowering workload and enhancing consistency. It enhances productiveness, hurries up decision-making, and supplies dependable responses throughout completely different sectors.
An AI-powered question decision system is beneficial in buyer help, the place it automates responses and improves buyer satisfaction. In gross sales and advertising and marketing, it supplies real-time product particulars and buyer insights. Industries like finance, healthcare, training, and e-commerce profit from automated question dealing with, making certain clean operations and higher person experiences.
Understanding the RAG Workflow
Earlier than diving into the implementation, let’s first perceive how a Retrieval-Augmented Era (RAG) system works.
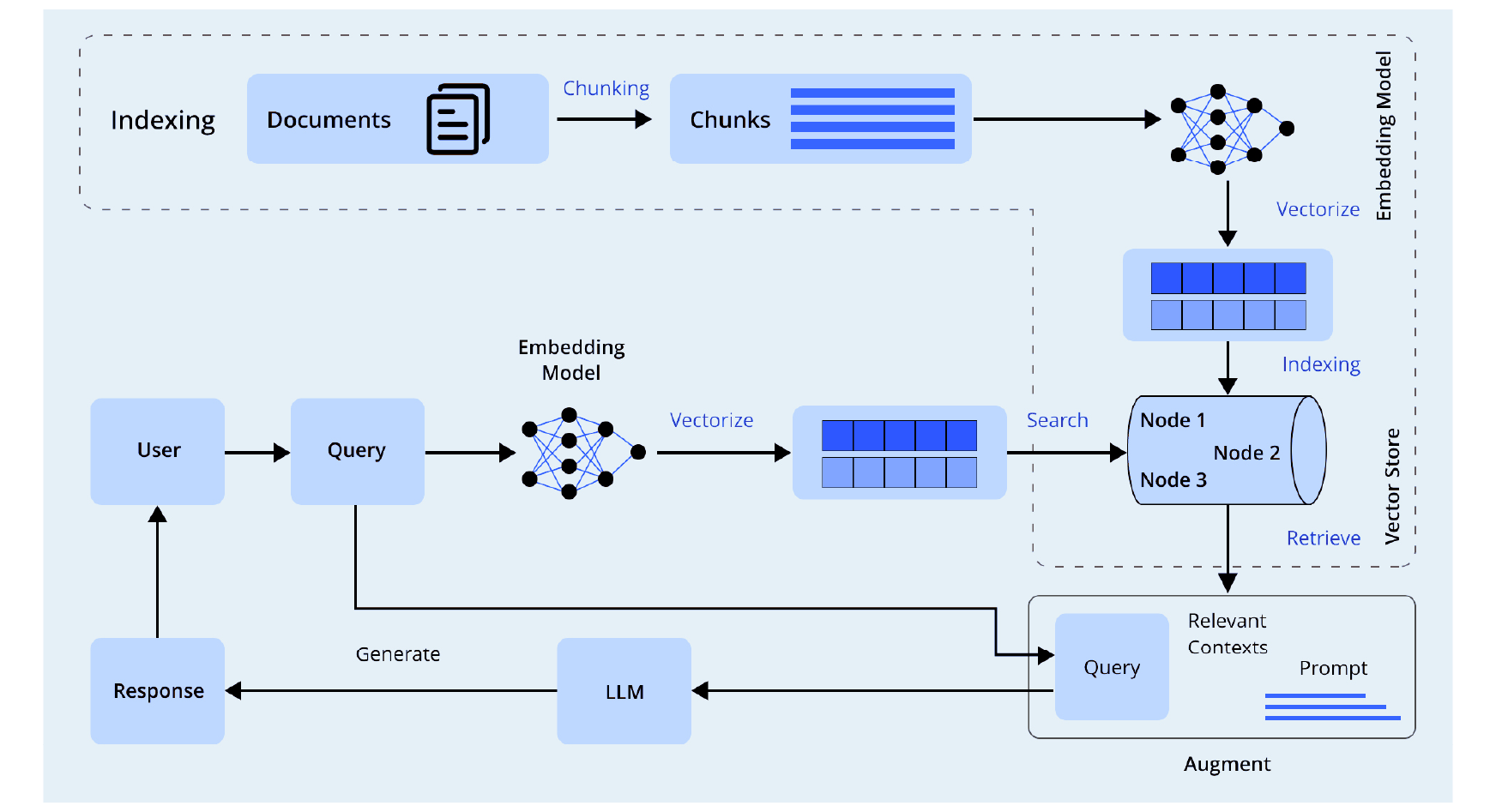
The structure consists of three key levels: Indexing, Retrieval, and Era.
1. Constructing a Vector Retailer (Doc Processing & Storage)
The system first processes and shops related paperwork to make them simply searchable. Right here’s how the indexing course of works:
- Paperwork & Chunking: Massive paperwork are damaged into smaller textual content chunks for environment friendly retrieval.
- Embedding Mannequin: These textual content chunks are transformed into vector representations utilizing an AI-based embedding mannequin.
- Vector Retailer: The vectorized information is listed and saved in a database (e.g., ChromaDB) for quick lookup.
2. Question Processing & Retrieval
When a person submits a question, the system retrieves related information earlier than producing a response. Listed below are the steps concerned in question processing and retrieval:
- Consumer Question Enter: The person submits a query or request.
- Vectorization: The question is transformed right into a numerical vector utilizing the embedding mannequin.
- Search & Retrieval: The system searches for essentially the most related chunks within the vector retailer and retrieves them.
3. Augmentation & Response Era
To generate a well-informed response, the system augments the question with retrieved information. Given under are the steps concerned in response technology.
- Increase Question: The retrieved doc chunks are mixed with the unique question.
- LLM Processing: A big language mannequin (LLM) generates a closing response utilizing each the question and the retrieved context.
- Remaining Response: The system supplies a factual and context-aware reply to the person.
Now that you know the way RAG programs work, let’s discover ways to construct a RAG-based question decision system.
Constructing a RAG-based Question Decision System
On this article, I’ll stroll you thru constructing a RAG-based Question Decision System that effectively solutions learner queries utilizing an AI agent. To maintain issues easy, I’ll exhibit a simplified model of the venture and clarify the way it works.
Deciding on the Proper Knowledge for Question Decision
Earlier than constructing a RAG-based question decision system, an important issue to contemplate is information – particularly, the sorts of information required for efficient retrieval. A well-structured information base is important, because the accuracy and relevance of responses depend upon the standard of the information out there. Beneath are the important thing information varieties that ought to be thought of for various functions:
- Buyer Help Knowledge: FAQs, troubleshooting guides, product manuals, and previous buyer interactions.
- Gross sales & Advertising and marketing Knowledge: Product catalogs, pricing particulars, competitor evaluation, and buyer inquiries.
- Inner Data Base: Firm insurance policies, coaching paperwork, and normal working procedures (SOPs).
- Monetary & Authorized Paperwork: Compliance pointers, monetary stories, and regulatory insurance policies.
- Consumer-Generated Content material: Discussion board discussions, chat logs, and suggestions varieties that present real-world person queries.
Deciding on the appropriate information sources was essential for our learner question decision system, to make sure correct and related responses. Initially, I experimented with several types of information to find out which offered the very best outcomes. First, I used PowerPoint slides (PPTs), however they didn’t yield complete solutions as anticipated. Subsequent, I included frequent queries, which improved response accuracy however lacked enough context. Then, I examined previous discussions, which helped in making responses extra related by leveraging earlier learner interactions. Nonetheless, the simplest strategy turned out to be utilizing subtitles from course movies, as they offered structured and detailed content material instantly associated to learner queries. This strategy helps in offering fast and related solutions, making it helpful for e-learning platforms and academic help programs.
Structuring the Question Decision System
Earlier than coding, it is very important construction the Question Decision System. One of the simplest ways to do that is by defining the important thing duties it must carry out.
The system will deal with three primary duties:
- Extract and retailer course content material from subtitles (SRT information).
- Retrieve related course supplies based mostly on learner queries.
- Use an AI-powered agent to generate structured responses.
To realize this, the system is split into three elements, every dealing with a selected perform. This ensures effectivity and scalability.
The system consists of:
- Subtitle Processing – Extracts textual content from SRT information, processes it, and shops embeddings in ChromaDB.
- Retrieval – Searches and retrieves related course supplies based mostly on learner queries.
- Question Answering Agent – Makes use of CrewAI to generate structured and correct responses.
Every element ensures environment friendly question decision, customized responses, and clean content material retrieval. Now that we have now our construction, let’s transfer on to implementation.
Implementation Steps
Now that we have now our construction, let’s transfer on to implementation.
1. Importing Libraries
To construct the AI-powered studying help system, we first have to import important libraries.
import pysrt
from langchain.text_splitter import RecursiveCharacterTextSplitter
from langchain.schema import Doc
from langchain.embeddings import OpenAIEmbeddings
from langchain.vectorstores import Chroma
from crewai import Agent, Activity, Crew
import pandas as pd
import ast
Let’s perceive these libraries.
- pysrt – For extracting textual content from SRT subtitle information.
- langchain.text_splitter.RecursiveCharacterTextSplitter – Splits massive textual content into smaller chunks for higher retrieval.
- langchain.schema.Doc – Represents structured textual content paperwork.
- langchain.embeddings.OpenAIEmbeddings – Converts textual content into numerical vectors for similarity searches.
- langchain.vectorstores.Chroma – Shops embeddings in a vector database for environment friendly retrieval.
- crewai (Agent, Activity, Crew) – Defines AI brokers that course of learner queries.
- pandas – Handles structured information within the type of DataFrames.
- ast – Helps in parsing string-based information buildings into Python objects.
- os – Gives system-level operations like studying atmosphere variables.
- tqdm – Shows progress bars throughout long-running duties.
2. Setting Up the Setting
To make use of OpenAI’s API for embeddings, we should load the API key and configure the mannequin settings.
Step 1: Learn the API key from a neighborhood textual content file.
with open('/house/janvi/Downloads/openai.txt', 'r') as file:
openai_api_key = file.learn()
Step 2: Retailer the API key as an atmosphere variable so it may be accessed by different elements.
os.environ['OPENAI_API_KEY'] = openai_api_key
Step3: Specify the OpenAI mannequin for use for processing embeddings.
os.environ["OPENAI_MODEL_NAME"] = 'gpt-4o-mini'
By establishing these configurations, we guarantee seamless integration with OpenAI’s API, permitting our system to course of and retailer embeddings effectively.
3. Extracting and Storing Subtitle Knowledge
Subtitles usually comprise precious insights from video lectures, making them a wealthy supply of structured content material for AI-based retrieval programs. Extracting and processing subtitle information successfully permits for environment friendly search and retrieval of related info when answering learner queries.
To protect instructional insights, we’re utilizing pysrt to learn and preprocess textual content from SRT information. This ensures the extracted content material is structured and prepared for additional processing and storage..
def extract_text_from_srt(srt_path):
"""Extracts textual content from an SRT subtitle file utilizing pysrt."""
subs = pysrt.open(srt_path)
textual content = " ".be a part of(sub.textual content for sub in subs)
return textual content
Since programs might have a number of subtitle information, we systematically manage and iterate by means of course supplies saved in predefined folders. This enables for seamless textual content extraction and additional processing.
# Outline course names and their respective folder paths
course_folders = {
"Introduction to Deep Studying utilizing PyTorch": "C:MCodeGAILearn_queriesSubtitle_Introduction_to_Deep_Learning_Using_Pytorch",
"Constructing Manufacturing-Prepared RAG programs utilizing LlamaIndex": "C:MCodeGAILearn_queriesSubtitle of Constructing Manufacturing-Prepared RAG programs utilizing LlamaIndex",
"Introduction to LangChain - Constructing Generative AI Apps & Brokers": "C:MCodeGAILearn_queriesSubtitle_introduction_to_langchain_using_agentic_ai"
}
# Dictionary to retailer course names and their respective .srt file paths
course_srt_files = {}
# Iterate by means of course folder mappings
for course, folder_path in course_folders.gadgets():
srt_files = []
# Stroll by means of the listing to seek out .srt information
for root, _, information in os.stroll(folder_path):
srt_files.prolong(os.path.be a part of(root, file) for file in information if file.endswith(".srt"))
# Add to dictionary if there are .srt information
if srt_files:
course_srt_files[course] = srt_files
This extracted textual content varieties the inspiration of our AI-driven studying help system, enabling superior retrieval and question decision.
Step 2: Storing Subtitles in ChromaDB
On this half, we are going to break down the method of storing course subtitles in ChromaDB, together with textual content chunking, embedding technology, persistence, and price estimation.
a. Persistent Listing for ChromaDB
The persist_directory is a folder path the place the saved information can be saved, permitting us to retain embeddings even after restarting this system. With out this, the database would reset after every execution.
persist_directory = "./subtitles_db"
ChromaDB is used as a vector database to retailer and retrieve embeddings effectively.
b. Splitting Textual content into Smaller Chunks
Massive paperwork (like total course subtitles) exceed token limits for embeddings. To deal with this, we use RecursiveCharacterTextSplitter to interrupt textual content into smaller, overlapping chunks to enhance search accuracy.
# Textual content splitter to interrupt paperwork into smaller chunks
text_splitter = RecursiveCharacterTextSplitter(chunk_size=1000, chunk_overlap=200)
Every chunk is 1,000 characters lengthy, making certain that the textual content is damaged into manageable items. To keep up context between chunks, 200 characters from the earlier chunk are included within the subsequent one. This overlap helps protect vital particulars and improves retrieval accuracy.
c. Initializing OpenAI Embeddings and ChromaDB Vector Retailer
We have to convert textual content into numerical vector representations for similarity search. OpenAI’s embeddings enable us to encode our course content material right into a format that may be searched effectively.
# Initialize OpenAI embeddings
embeddings = OpenAIEmbeddings(openai_api_key=openai_api_key)
Right here, OpenAIEmbeddings() initializes the embedding mannequin utilizing our OpenAI API key (openai_api_key). This ensures that each textual content chunk will get transformed right into a high-dimensional vector illustration.
d. Initializing ChromaDB
Now, we retailer these vector embeddings in ChromaDB.
# Initialize Chroma vectorstore with persistent listing
vectorstore = Chroma(
collection_name="course_materials",
embedding_function=embeddings,
persist_directory=persist_directory
)
The collection_name=”course_materials” creates a devoted assortment in ChromaDB to prepare all course-related embeddings. The embedding_function=embeddings specifies OpenAI embeddings for changing textual content into numerical vectors. The persist_directory=persist_directory ensures that each one saved embeddings stay out there in ./subtitles_db/, even after restarting this system.
Step 3: Estimating Price of Storing Course Knowledge
Earlier than including paperwork to the vector database, it’s important to estimate the price of token utilization. Since OpenAI fees per 1,000 tokens, we calculate the anticipated value to handle bills effectively.
a. Defining Pricing Parameters
Since OpenAI fees per 1,000 tokens, we estimate the fee earlier than including paperwork.
import time
# OpenAI Pricing (modify based mostly on the mannequin getting used)
COST_PER_1K_TOKENS = 0.0001 # Price per 1K tokens for 'text-embedding-ada-002'
TOKENS_PER_CHUNK_ESTIMATE = 750 # Approximate tokens per 1000-character chunk
# Observe complete tokens and price
total_tokens = 0
total_cost = 0
# Begin timing
start_time = time.time()
The COST_PER_1K_TOKENS = 0.0001 defines the fee per 1,000 tokens when utilizing OpenAI embeddings. The TOKENS_PER_CHUNK_ESTIMATE = 750 estimates that every 1,000-character chunk comprises about 750 tokens. The total_tokens and total_cost variables monitor the whole processed information and price incurred throughout execution. The start_time variable information the beginning time to measure how lengthy the method takes.
b. Checking and Including Programs to ChromaDB
We wish to keep away from reprocessing programs which are already saved within the vector database. So for that we’re querying ChromaDB to test if the course already exists. If the course shouldn’t be discovered, we extract and retailer its subtitle information.
# Add new programs to the vectorstore if they do not exist already
for course, srt_list in course_srt_files.gadgets():
# Examine if the course already exists within the vectorstore
existing_docs = vectorstore._collection.get(the place={"course": course})
if not existing_docs['ids']:
# Course not discovered, add it
srt_texts = [extract_text_from_srt(srt) for srt in srt_list]
course_text = "nnnn".be a part of(srt_texts) # Be part of SRT texts with 4 new strains
doc = Doc(page_content=course_text, metadata={"course": course})
chunks = text_splitter.split_documents([doc])
The subtitles are extracted utilizing the extract_text_from_srt() perform. A number of subtitle information are then joined collectively utilizing nnnn to enhance readability. A Doc object is created, storing the complete subtitle textual content together with its metadata. Lastly, the textual content is break up into smaller chunks utilizing text_splitter.split_documents() for environment friendly processing and retrieval.
c. Estimating Token Utilization and Price
Earlier than including the chunks to ChromaDB, we estimate the fee.
# Estimate value earlier than including paperwork
chunk_count = len(chunks)
batch_tokens = chunk_count * TOKENS_PER_CHUNK_ESTIMATE
batch_cost = (batch_tokens / 1000) * COST_PER_1K_TOKENS
total_tokens += batch_tokens
total_cost += batch_cost
The chunk_count represents the variety of chunks generated after splitting the textual content. The batch_tokens estimates the whole variety of tokens based mostly on the chunk depend. The batch_cost calculates the estimated value for processing the present course. The total_tokens and total_cost accumulate values throughout all programs to trace total processing and bills.
d. Including Chunks to ChromaDB
vectorstore.add_documents(chunks)
print(f"Added course: {course} (Chunks: {chunk_count}, Price: ${batch_cost:.4f})")
else:
print(f"Course already exists: {course}")
The processed chunks are saved in ChromaDB for environment friendly retrieval. A message is displayed, indicating the variety of chunks added and the estimated processing value.
As soon as all programs are processed, we calculate and show the ultimate outcomes.
# Finish timing
end_time = time.time()
# Show value and time
print(f"nCourse Embeddings Replace Accomplished! 🚀")
print(f"Whole Chunks Processed: {total_tokens // TOKENS_PER_CHUNK_ESTIMATE}")
print(f"Estimated Whole Tokens: {total_tokens}")
print(f"Estimated Price: ${total_cost:.4f}")
print(f"Whole Time Taken: {end_time - start_time:.2f} seconds")
The whole processing time is calculated utilizing (end_time – start_time). The system then shows the variety of chunks processed, the estimated token utilization, and the general value. Lastly, it supplies a abstract of the complete embedding course of.
Output:

From the output, we will see {that a} complete of 739 chunks had been processed in 10 seconds, with an estimated value of $0.0554.
4. Querying and Responding to Learner Queries
As soon as the subtitles are saved in ChromaDB, the system wants a technique to retrieve related content material when a learner submits a question. This retrieval course of is dealt with utilizing similarity search, which identifies saved textual content segments that are most related to the enter question.
The way it Works:
- Question Enter: The learner submits a query associated to the course.
- Filtering by Course: The system ensures that retrieval is restricted to the related course materials.
- Similarity Search in ChromaDB: The question is transformed into an embedding, and ChromaDB retrieves essentially the most comparable saved textual content chunks.
- Returning the Prime Outcomes: The system selects the highest three most related textual content segments.
- Formatting the Output: The retrieved textual content is formatted and offered as context for additional processing.
# Outline retrieval instrument with metadata filtering
def retrieve_course_materials(question: str, course = course):
"""Retrieves course supplies filtered by course identify."""
filter_dict = {"course": course}
outcomes = vectorstore.similarity_search(question, ok=3, filter=filter_dict)
return "nn".be a part of([doc.page_content for doc in results])
Instance queries:
course_name = "Introduction to Deep Studying utilizing PyTorch"
query = "What's gradient descent?"
context = retrieve_course_materials(question=query, course= course_name)
print(context)
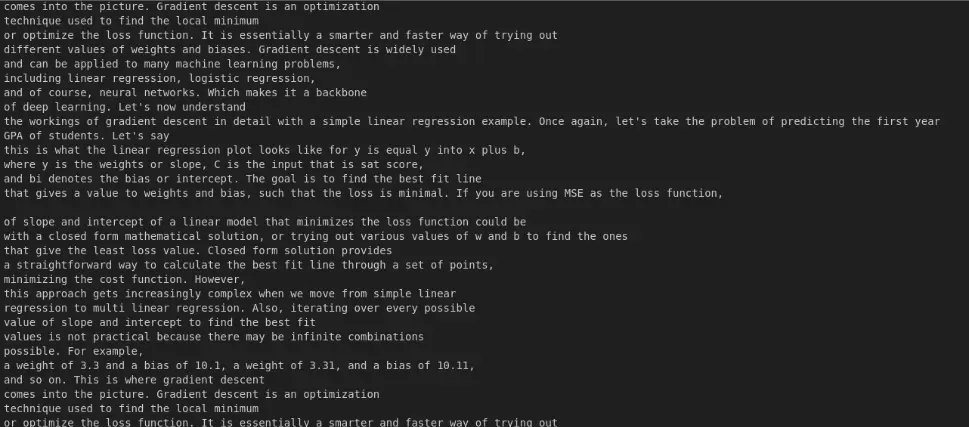
The output consists of the retrieved content material from ChromaDB, filtered by course identify and query, utilizing similarity search to seek out essentially the most related info.
Why is Similarity Search Used?
- Semantic Understanding: Not like key phrase searches, similarity search finds textual content semantically associated to the question.
- Environment friendly Retrieval: As a substitute of scanning total paperwork, the system retrieves solely essentially the most related components.
- Improved Reply High quality: By filtering by course and rating outcomes by relevance, learners obtain extremely focused content material.
This mechanism ensures that when a learner submits a query, they obtain related and contextually correct info from saved course supplies.
5. Implementing the AI Question Answering Agent
As soon as related course materials is retrieved from ChromaDB, the following step is to make use of an AI-powered agent to formulate significant responses to learner queries. CrewAI is used to outline an clever agent accountable for analyzing queries and producing well-structured responses.
Now, let’s see the way it works.
Step 1: Defining the Agent
The question answering agent is created with a transparent function and backstory to information its conduct when responding to learner queries.
# Outline the agent with a well-structured function and backstory
query_answer_agent = Agent(
function = "Studying Help Specialist",
purpose = "You assist learners with their queries with the absolute best response",
backstory = """You lead the Learners Question decision division of
an Ed tech firm focussed on self paced programs on subjects associated to
Knowledge Science, Machine Studying and Generative AI. You reply to learner
queries associated to course content material, assignments, technical and administrative points.
You might be well mannered, diplomatic and take possession of issues which could possibly be
imporved in your oragnisation.
""",
verbose = False,
)
Let’s perceive what is going on within the code block. Firstly, we’re offering the function as Studying Help Specialist for the reason that agent acts as a digital tutor that solutions scholar queries. Then, we outline the purpose, making certain that the agent prioritizes accuracy and readability in its responses. Lastly, we set verbose=False, which retains the execution silent except debugging is required. This well-defined agent function ensures that responses are useful, structured, and aligned with the tutorial platform’s tone.
Step 2: Defining the Activity
After defining the agent, we have to assign it a job
query_answering_task = Activity(
description= """
Reply the learner queries to the very best of your talents. Attempt to maintain your response concise with lower than 100 phrases.
Right here is the question: {question}
Right here is comparable content material from the course extracted from subtitles, which it is best to use solely when required: {relevant_content} .
Since this content material is extracted from course subtitles, there could also be spelling errors, be certain to right these, whereas utilizing this info in your response.
There could also be some earlier dialogue with the learner on this thread. Right here is the python record of previous discussions: {thread} .
On this thread, the content material which begins with 'learner' is the query by the scholar and the content material which begins with 'help'
is the response given by you. Use this previous dialogue appropriatly to return with an important reply.
That is the complete identify of the learner: {learner_name}
Deal with every learner by their first identify, in case you are unsure what the primary identify is, merely begin with Hello.
Additionally point out some applicable and inspiring comforting strains on the finish of the reponse, like "hope you discovered this useful",
"I hope this info is beneficial. Sustain the nice work!", "Glad to help! Be happy to succeed in out anytime." and so forth.
If you're unsure in regards to the reply point out - "Sorry, I'm not positive about this, I'll get again to you"
""",
expected_output = "A crisp correct response to the question",
agent=query_answer_agent)
Let’s break down the duty offered to the AI agent. The question dealing with includes processing {question}, which represents the learner’s query. The response ought to be concise (beneath 100 phrases) and correct. When utilizing course content material, {relevant_content} is extracted from subtitles saved in ChromaDB, and the AI should right any spelling errors earlier than together with the content material in its response.
If previous discussions exist, {thread} helps preserve continuity. Learner queries begin with “learner”, whereas previous responses start with “help”, permitting the agent to offer context-aware solutions. Personalization is achieved utilizing {learner_name}—the agent addresses college students by their first identify or defaults to “Hello” if unsure.
To make responses extra partaking, the AI provides a optimistic closing assertion, similar to “Hope you discovered this useful!” or “Be happy to succeed in out anytime.” If the AI is uncertain about a solution, it explicitly states: “Sorry, I’m not positive about this, I’ll get again to you.” This strategy ensures politeness, readability, and a structured response format, enhancing learner engagement and belief.
Step 3: Initializing the CrewAI Occasion
Now that we have now each the agent and the duty, we initialize CrewAI, which allows the agent to course of queries dynamically.
# Create the Crew
response_crew = Crew(
brokers=[query_answer_agent],
duties=[query_answering_task],
verbose=False
)
The brokers=[query_answer_agent] parameter provides the Studying Help Specialist agent to the crew. The duties=[query_answering_task] assigns the question answering job to this agent. Setting verbose=False retains the output minimal except debugging is required. CrewAI allows the system to course of a number of learner queries concurrently, making it scalable and environment friendly for dynamic question dealing with.
Why Use CrewAI for Question Answering?
- Structured Responses: Ensures that every response is well-organized and informative.
- Context Consciousness: Makes use of retrieved course materials and previous discussions to enhance response high quality.
- Scalability: Can deal with a number of queries dynamically by processing them as duties inside CrewAI.
- Effectivity: Reduces response time by streamlining the question decision workflow.
By implementing this AI-powered answering system, learners obtain well-informed responses tailor-made to their particular queries.
Step 4: Producing Responses for A number of Learner’s Queries
As soon as the AI agent is about up, it must dynamically course of learner queries saved in a structured dataset.
The under code processes learner queries saved in a CSV file and generates responses utilizing an AI agent. It first masses the dataset containing learner queries, course particulars, and dialogue threads. The reply_to_query perform extracts related particulars just like the learner’s identify, course identify, and present question. If earlier discussions exist, they’re retrieved for context. If the question comprises a picture, it’s skipped. The perform then fetches associated course supplies from ChromaDB and sends the question, related content material, and previous discussions to the AI agent for producing a structured response.
df = pd.read_csv(filepath_or_buffer="C:MCodeGAILearn_queries/filtered_data_top3_courses.csv")
def reply_to_query(df_new=df_new, index=1):
learner_name = df_new.iloc[index]["thread_starter"]
course_name = df_new.iloc[index]["course"]
if df_new.iloc[index]['number_of_replies']>1:
thread = ast.literal_eval(df_new.iloc[index]["modified_thread"])
else:
thread = []
query = df_new.iloc[index]["current_query"]
if df_new.iloc[index]['has_image'] == True:
return " "
context = retrieve_course_materials(question = query , course=course_name)
response_result = response_crew.kickoff(inputs={"question": query, "relevant_content": context, "thread": thread, "learner_name": learner_name})
print('Q: ', query)
print('n')
print('A: ', response_result)
print('nn')
Testing the perform, it’s executed for one question (index=1)
reply_to_query(df, index=1)
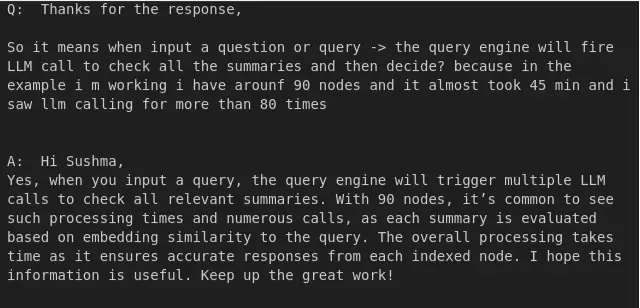
From this we will see that it really works superb only for one index.
Now, iterating by means of all queries, processing every one whereas dealing with potential errors. This ensures environment friendly automation of question decision, permitting a number of learner queries to be processed dynamically.
for i in vary(len(df)):
strive:
reply_to_query(df, index=i)
besides:
print("Error in index quantity: ", i)
proceed
Why is This Step Essential?
- Automates Question Processing: The system can deal with a number of learner queries effectively.
- Ensures Contextual Relevance: Responses are generated based mostly on retrieved course supplies and previous discussions.
- Scalability: The tactic permits the AI agent to course of and reply to hundreds of queries dynamically.
- Improved Studying Help: Learners obtain customized, data-driven responses to their queries.
This step ensures that each learner question is analyzed, contextualized, and answered successfully, enhancing the general studying expertise.
Output:
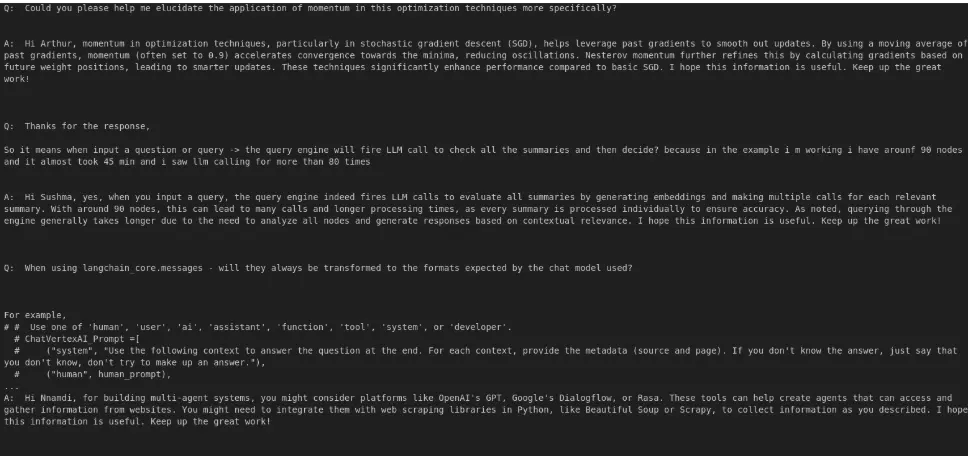
From the output we will see that the method of replying to the question has turn into automated adopted by query after which reply.
Future Enhancements
To improve the RAG-Primarily based Question Decision System, a number of enhancements might be made:
- Frequent Questions and Their Options: Implementing a structured FAQ system throughout the question decision framework will assist in offering on the spot solutions to often requested questions, lowering dependency on stay help.
- Picture Processing Skill: Including the aptitude to research and extract related info from pictures (similar to screenshots, charts, or scanned paperwork) will improve the system’s versatility, making it extra helpful in instructional and buyer help domains.
- Enhancing the Picture Column Boolean: Refining the logic behind the picture column detection to accurately determine and course of image-based queries with better accuracy.
- Semantic Chunking and Completely different Chunking Methods: Experimenting with numerous chunking methods, similar to semantic chunking, fixed-length segmentation, and hybrid approaches, can enhance retrieval accuracy and contextual understanding of responses.
Conclusion
This RAG-Primarily based Question Decision System leverages LangChain, ChromaDB, and CrewAI to automate learner help effectively. It extracts subtitles, shops them as embeddings in ChromaDB, and retrieves related content material utilizing similarity search. A CrewAI agent processes queries, references previous discussions, and generates structured responses, making certain accuracy and personalization.
The system enhances scalability, retrieval effectivity, and response high quality, making self-paced studying extra interactive. Future enhancements embody multi-modal help, higher retrieval optimization, and enhanced response technology. By automating question decision, this technique streamlines studying help, offering learners with sooner, context-aware responses and enhancing total engagement.
Steadily Requested Questions
A. LangChain is a framework for constructing functions powered by language fashions (LLMs). It helps in processing, retrieving, and producing responses from text-based information. On this venture, LangChain is used for splitting textual content into chunks, producing embeddings, and retrieving course supplies effectively.
A. ChromaDB is a vector database designed for storing and retrieving embeddings. It converts course supplies into numerical representations, permitting similarity-based searches to seek out related content material when a learner submits a question.
A. CrewAI allows the creation of AI brokers that deal with duties dynamically. On this venture, it powers a Studying Help Specialist agent that retrieves course supplies, processes previous discussions, and generates structured responses for learner queries.
A. OpenAI embeddings convert textual content into numerical vectors, making it simpler to carry out similarity searches. This helps in effectively retrieving related course supplies based mostly on a learner’s question.
A. The system makes use of pysrt to extract textual content from subtitle (SRT) information. The extracted content material is then chunked, embedded utilizing OpenAI embeddings, and saved in ChromaDB for retrieval when wanted.
A. Sure, the system is scalable and may course of a number of learner queries dynamically utilizing CrewAI’s job administration. This ensures fast and environment friendly responses.
A. Future enhancements embody multi-modal help for pictures and movies, higher retrieval optimization, and improved response technology methods to offer much more correct and contextual solutions.
Login to proceed studying and revel in expert-curated content material.