With the discharge of iOS 18, Apple has unveiled a collection of thrilling options beneath the Apple Intelligence umbrella, and one standout is the ImagePlayground
framework. This highly effective API empowers builders to generate pictures from textual content descriptions utilizing AI, opening up a world of artistic prospects for iOS apps. Whether or not you’re constructing a design instrument, a storytelling app, or simply need to add some aptitude to your UI, ImagePlayground makes it seamless to combine AI-driven picture era.
On this tutorial, we’ll stroll you thru constructing a easy app utilizing SwiftUI and the ImagePlayground
framework. Our app will let customers sort an outline—like “a serene seashore at sundown”—and generate a corresponding picture with a faucet. Designed for builders with some iOS expertise, this information assumes you’re accustomed to Swift, SwiftUI, and Xcode fundamentals. Able to dive into iOS 18’s picture era capabilities?
Let’s get began!
Stipulations
Earlier than we get began, be sure to’ve obtained a couple of issues prepared:
- Machine: Picture Playground is supported on iPhone 15 Professional, iPhone 15 Professional Max, and all iPhone 16 fashions.
- iOS Model: Your gadget have to be operating iOS 18.1 or later.
- Xcode: You’ll want Xcode 16 or later to construct the app.
- Apple Intelligence: Make sure that Apple Intelligence is enabled in your gadget. You possibly can verify this in Settings > Apple Intelligence & Siri. If prompted, request entry to Apple Intelligence options.
Establishing the Xcode Challenge
First, let’s start by creating a brand new Xcode undertaking named AIImageGeneration
utilizing the iOS app template. Ensure you select SwiftUI because the UI framework. Additionally, the minimal deployment model is about to 18.1 (or later). The ImagePlayground
framework is just obtainable on iOS 18.1 or up.
Utilizing ImagePlaygroundSheet
Have you ever tried Picture Playground app in iOS 18 earlier than? The app leverages Apple Intelligence to create pictures primarily based on consumer inputs, resembling textual content descriptions. Whereas Playground is an impartial app on iOS, builders can carry this performance into your apps utilizing ImagePlaygroundSheet
, a SwiftUI view modifier that presents the picture era interface.
Let’s swap over to the Xcode undertaking and see how the sheet works. Within the ContentView.swift
file, add the next import assertion:
import ImagePlayground
The ImagePlaygroundSheet
view is included within the ImagePlayground
framework. For the ContentView
struct, replace it like beneath:
struct ContentView: View {
@Setting(.supportsImagePlayground) non-public var supportsImagePlayground
@State non-public var showImagePlayground: Bool = false
@State non-public var generatedImageURL: URL?
var physique: some View {
if supportsImagePlayground {
if let generatedImageURL {
AsyncImage(url: generatedImageURL) { picture in
picture
.resizable()
.scaledToFill()
} placeholder: {
Shade.purple.opacity(0.1)
}
.padding()
}
Button {
showImagePlayground.toggle()
} label: {
Textual content("Generate pictures")
}
.buttonStyle(.borderedProminent)
.controlSize(.massive)
.tint(.purple)
.imagePlaygroundSheet(isPresented: $showImagePlayground) { url in
generatedImageURL = url
}
.padding()
} else {
ContentUnavailableView("Not Supported", systemImage: "exclamationmark.triangle", description: Textual content("This gadget doesn't assist Picture Playground. Please use a tool that helps Picture Playground to view this instance."))
}
}
}
Not all iOS units have Apple Intelligence enabled. That’s why it’s essential to do a fundamental verify to see if ImagePlayground
is supported on the gadget. The supportsImagePlayground
property makes use of SwiftUI’s setting system to verify if the gadget can use Picture Playground. If the gadget doesn’t assist it, we merely present a “Not Supported” message on the display screen.
For units that do assist it, the demo app shows a “Generate Pictures” button. The best approach so as to add Picture Playground to your app is by utilizing the imagePlaygroundSheet
modifier. We use the showImagePlayground
property to open or shut the playground sheet. After the consumer creates a picture in Picture Playground, the system saves the picture file in a brief location and provides again the picture URL. This URL is then assigned to the generatedImageURL
variable.
With the picture URL prepared, we use the AsyncImage
view to show the picture on the display screen.
Run the app and take a look at it in your iPhone. Faucet the “Generate Picture” button to open the Picture Playground sheet. Enter an outline for the picture, and let Apple Intelligence create it for you. As soon as it’s achieved, shut the sheet, and the generated picture ought to seem within the app.
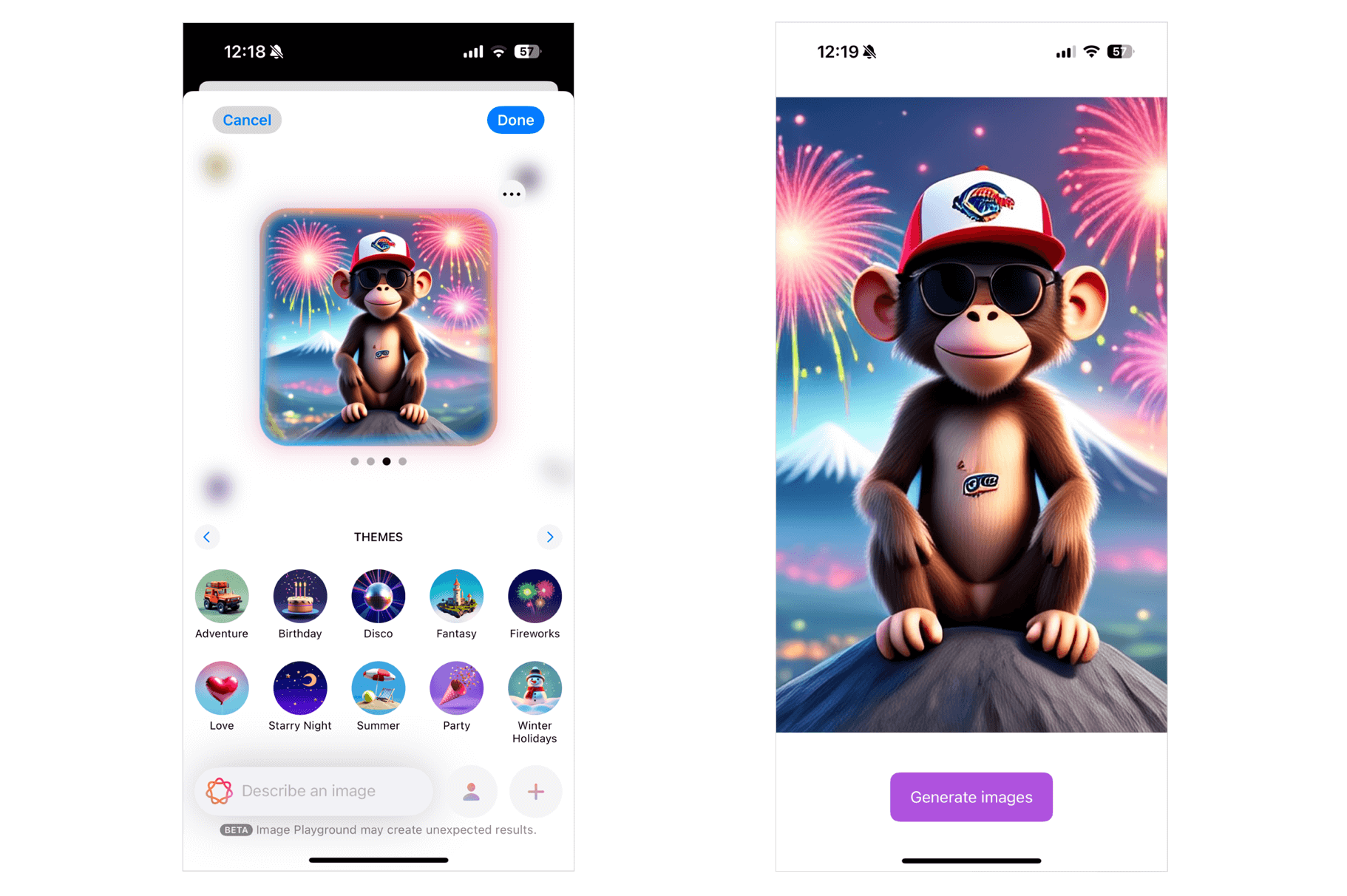
Working with Ideas
Beforehand, I confirmed you the essential approach of utilizing the imagePlaygroundSheet
modifier. The modifier supplies a lot of parameters for builders to customise the combination. For instance, we are able to create our personal textual content subject to seize the outline of the picture.
In ContentView
, replace the code like beneath:
struct ContentView: View {
@Setting(.supportsImagePlayground) non-public var supportsImagePlayground
@State non-public var showImagePlayground: Bool = false
@State non-public var generatedImageURL: URL?
@State non-public var description: String = ""
var physique: some View {
if supportsImagePlayground {
if let generatedImageURL {
AsyncImage(url: generatedImageURL) { picture in
picture
.resizable()
.scaledToFill()
} placeholder: {
Shade.purple.opacity(0.1)
}
.padding()
} else {
Textual content("Kind your picture description to create a picture...")
.font(.system(.title, design: .rounded, weight: .medium))
.multilineTextAlignment(.heart)
.body(maxWidth: .infinity, maxHeight: .infinity)
}
Spacer()
HStack {
TextField("Enter your textual content...", textual content: $description)
.padding()
.background(
RoundedRectangle(cornerRadius: 12)
.fill(.white)
)
.overlay(
RoundedRectangle(cornerRadius: 12)
.stroke(Shade.grey.opacity(0.2), lineWidth: 1)
)
.font(.system(measurement: 16, weight: .common, design: .rounded))
Button {
showImagePlayground.toggle()
} label: {
Textual content("Generate pictures")
}
.buttonStyle(.borderedProminent)
.controlSize(.common)
.tint(.purple)
.imagePlaygroundSheet(isPresented: $showImagePlayground,
idea: description
) { url in
generatedImageURL = url
}
.padding()
}
.padding(.horizontal)
} else {
ContentUnavailableView("Not Supported", systemImage: "exclamationmark.triangle", description: Textual content("This gadget doesn't assist Picture Playground. Please use a tool that helps Picture Playground to view this instance."))
}
}
}
We added a brand new textual content subject the place customers can immediately enter a picture description. The imagePlaygroundSheet
modifier has been up to date with a brand new parameter known as idea
. This parameter accepts the picture description and passes it to the creation UI to generate the picture.
.imagePlaygroundSheet(isPresented: $showImagePlayground,
idea: description
) { url in
generatedImageURL = url
}
The idea
parameter works greatest for brief descriptions. If you wish to enable customers to enter an extended paragraph, it’s higher to make use of the ideas
parameter, which takes an array of ImagePlaygroundConcept
. Under is an instance of how the code might be rewritten utilizing the ideas
parameter:
.imagePlaygroundSheet(isPresented: $showImagePlayground,
ideas: [ .text(description) ]
) { url in
generatedImageURL = url
}
The textual content
operate creates a playground idea by processing a brief description of the picture. For longer textual content, you should utilize the extracted(from:title:)
API, which lets the system analyze the textual content and extract key ideas to information the picture creation course of.
Including a Supply Picture
The imagePlaygroundSheet
modifier additionally helps including a supply picture, which acts as the start line for picture era. Right here is an instance:
.imagePlaygroundSheet(isPresented: $showImagePlayground,
ideas: [.text(description)],
sourceImage: Picture("gorilla")
) { url in
generatedImageURL = url
}
.padding()
You possibly can both use the sourceImage
or sourceImageURL
parameter to embed the picture.
Abstract
On this tutorial, we explored the potential of the ImagePlayground
framework in iOS 18, showcasing how builders can harness its AI-driven picture era capabilities to create dynamic and visually partaking experiences. By combining the facility of SwiftUI with ImagePlayground
, we demonstrated how easy it’s to show textual content descriptions into beautiful visuals.
Now it’s your flip to discover this modern framework and unlock its full potential in your personal tasks. I am desirous to see what new AI-related frameworks Apple will introduce subsequent!