At the moment, most functions can ship a whole lot of requests for a single web page.
For instance, my Twitter residence web page sends round 300 requests, and an Amazon
product particulars web page sends round 600 requests. A few of them are for static
belongings (JavaScript, CSS, font recordsdata, icons, and so forth.), however there are nonetheless
round 100 requests for async information fetching – both for timelines, associates,
or product suggestions, in addition to analytics occasions. That’s fairly a
lot.
The principle purpose a web page might include so many requests is to enhance
efficiency and consumer expertise, particularly to make the appliance really feel
sooner to the top customers. The period of clean pages taking 5 seconds to load is
lengthy gone. In trendy internet functions, customers usually see a fundamental web page with
fashion and different components in lower than a second, with further items
loading progressively.
Take the Amazon product element web page for instance. The navigation and high
bar seem virtually instantly, adopted by the product photos, temporary, and
descriptions. Then, as you scroll, “Sponsored” content material, rankings,
suggestions, view histories, and extra seem.Typically, a consumer solely desires a
fast look or to match merchandise (and examine availability), making
sections like “Clients who purchased this merchandise additionally purchased” much less important and
appropriate for loading by way of separate requests.
Breaking down the content material into smaller items and loading them in
parallel is an efficient technique, nevertheless it’s removed from sufficient in massive
functions. There are numerous different features to think about on the subject of
fetch information accurately and effectively. Information fetching is a chellenging, not
solely as a result of the character of async programming does not match our linear mindset,
and there are such a lot of elements could cause a community name to fail, but in addition
there are too many not-obvious instances to think about beneath the hood (information
format, safety, cache, token expiry, and so forth.).
On this article, I wish to focus on some frequent issues and
patterns it is best to take into account on the subject of fetching information in your frontend
functions.
We’ll start with the Asynchronous State Handler sample, which decouples
information fetching from the UI, streamlining your software structure. Subsequent,
we’ll delve into Fallback Markup, enhancing the intuitiveness of your information
fetching logic. To speed up the preliminary information loading course of, we’ll
discover methods for avoiding Request
Waterfall and implementing Parallel Information Fetching. Our dialogue will then cowl Code Splitting to defer
loading non-critical software components and Prefetching information based mostly on consumer
interactions to raise the consumer expertise.
I consider discussing these ideas by a simple instance is
the very best method. I purpose to start out merely after which introduce extra complexity
in a manageable approach. I additionally plan to maintain code snippets, significantly for
styling (I am using TailwindCSS for the UI, which can lead to prolonged
snippets in a React part), to a minimal. For these within the
full particulars, I’ve made them out there on this
repository.
Developments are additionally taking place on the server aspect, with strategies like
Streaming Server-Aspect Rendering and Server Elements gaining traction in
varied frameworks. Moreover, a lot of experimental strategies are
rising. Nonetheless, these matters, whereas doubtlessly simply as essential, could be
explored in a future article. For now, this dialogue will focus
solely on front-end information fetching patterns.
It is vital to notice that the strategies we’re protecting will not be
unique to React or any particular frontend framework or library. I’ve
chosen React for illustration functions on account of my in depth expertise with
it lately. Nonetheless, ideas like Code Splitting,
Prefetching are
relevant throughout frameworks like Angular or Vue.js. The examples I will share
are frequent situations you would possibly encounter in frontend improvement, regardless
of the framework you utilize.
That mentioned, let’s dive into the instance we’re going to make use of all through the
article, a Profile
display screen of a Single-Web page Software. It is a typical
software you might need used earlier than, or not less than the state of affairs is typical.
We have to fetch information from server aspect after which at frontend to construct the UI
dynamically with JavaScript.
Introducing the appliance
To start with, on Profile
we’ll present the consumer’s temporary (together with
identify, avatar, and a brief description), after which we additionally wish to present
their connections (much like followers on Twitter or LinkedIn
connections). We’ll have to fetch consumer and their connections information from
distant service, after which assembling these information with UI on the display screen.
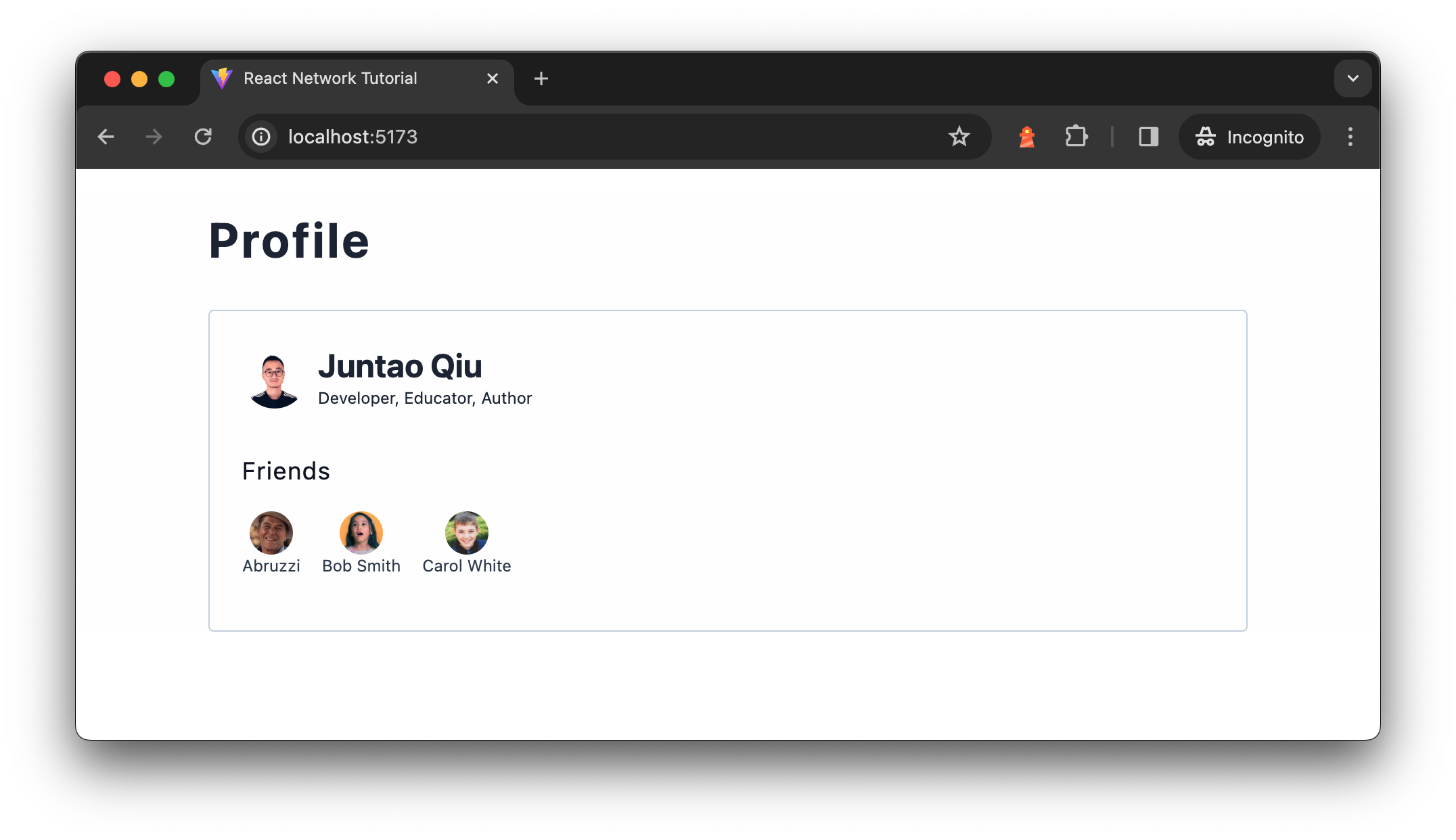
Determine 1: Profile display screen
The information are from two separate API calls, the consumer temporary API
/customers/
returns consumer temporary for a given consumer id, which is an easy
object described as follows:
{ "id": "u1", "identify": "Juntao Qiu", "bio": "Developer, Educator, Writer", "pursuits": [ "Technology", "Outdoors", "Travel" ] }
And the pal API /customers/
endpoint returns an inventory of
associates for a given consumer, every checklist merchandise within the response is identical as
the above consumer information. The rationale now we have two endpoints as a substitute of returning
a associates
part of the consumer API is that there are instances the place one
might have too many associates (say 1,000), however most individuals do not have many.
This in-balance information construction may be fairly tough, particularly after we
have to paginate. The purpose right here is that there are instances we have to deal
with a number of community requests.
A quick introduction to related React ideas
As this text leverages React as an instance varied patterns, I do
not assume you understand a lot about React. Slightly than anticipating you to spend so much
of time looking for the best components within the React documentation, I’ll
briefly introduce these ideas we’ll make the most of all through this
article. In case you already perceive what React elements are, and the
use of the
useState
and useEffect
hooks, chances are you’ll
use this hyperlink to skip forward to the following
part.
For these searching for a extra thorough tutorial, the new React documentation is a wonderful
useful resource.
What’s a React Element?
In React, elements are the basic constructing blocks. To place it
merely, a React part is a perform that returns a chunk of UI,
which may be as easy as a fraction of HTML. Take into account the
creation of a part that renders a navigation bar:
import React from 'react'; perform Navigation() { return ( ); }
At first look, the combination of JavaScript with HTML tags might sound
unusual (it is known as JSX, a syntax extension to JavaScript. For these
utilizing TypeScript, an identical syntax known as TSX is used). To make this
code practical, a compiler is required to translate the JSX into legitimate
JavaScript code. After being compiled by Babel,
the code would roughly translate to the next:
perform Navigation() { return React.createElement( "nav", null, React.createElement( "ol", null, React.createElement("li", null, "Dwelling"), React.createElement("li", null, "Blogs"), React.createElement("li", null, "Books") ) ); }
Be aware right here the translated code has a perform known as
React.createElement
, which is a foundational perform in
React for creating components. JSX written in React elements is compiled
all the way down to React.createElement
calls behind the scenes.
The fundamental syntax of React.createElement
is:
React.createElement(kind, [props], [...children])
kind
: A string (e.g., ‘div’, ‘span’) indicating the kind of
DOM node to create, or a React part (class or practical) for
extra refined constructions.props
: An object containing properties handed to the
factor or part, together with occasion handlers, types, and attributes
likeclassName
andid
.kids
: These optionally available arguments may be further
React.createElement
calls, strings, numbers, or any combine
thereof, representing the factor’s kids.
For example, a easy factor may be created with
React.createElement
as follows:
React.createElement('div', { className: 'greeting' }, 'Good day, world!');
That is analogous to the JSX model:
Good day, world!
Beneath the floor, React invokes the native DOM API (e.g.,
doc.createElement("ol")
) to generate DOM components as vital.
You possibly can then assemble your customized elements right into a tree, much like
HTML code:
import React from 'react'; import Navigation from './Navigation.tsx'; import Content material from './Content material.tsx'; import Sidebar from './Sidebar.tsx'; import ProductList from './ProductList.tsx'; perform App() { return; } perform Web page() { return ; }
In the end, your software requires a root node to mount to, at
which level React assumes management and manages subsequent renders and
re-renders:
import ReactDOM from "react-dom/shopper"; import App from "./App.tsx"; const root = ReactDOM.createRoot(doc.getElementById('root')); root.render();
Producing Dynamic Content material with JSX
The preliminary instance demonstrates a simple use case, however
let’s discover how we will create content material dynamically. For example, how
can we generate an inventory of knowledge dynamically? In React, as illustrated
earlier, a part is basically a perform, enabling us to move
parameters to it.
import React from 'react'; perform Navigation({ nav }) { return ( ); }
On this modified Navigation
part, we anticipate the
parameter to be an array of strings. We make the most of the map
perform to iterate over every merchandise, reworking them into
components. The curly braces {}
signify
that the enclosed JavaScript expression must be evaluated and
rendered. For these curious concerning the compiled model of this dynamic
content material dealing with:
perform Navigation(props) { var nav = props.nav; return React.createElement( "nav", null, React.createElement( "ol", null, nav.map(perform(merchandise) { return React.createElement("li", { key: merchandise }, merchandise); }) ) ); }
As an alternative of invoking Navigation
as a daily perform,
using JSX syntax renders the part invocation extra akin to
writing markup, enhancing readability:
// As an alternative of this Navigation(["Home", "Blogs", "Books"]) // We do that
Components in React can receive diverse data, known as props, to
modify their behavior, much like passing arguments into a function (the
distinction lies in using JSX syntax, making the code more familiar and
readable to those with HTML knowledge, which aligns well with the skill
set of most frontend developers).
import React from 'react'; import Checkbox from './Checkbox'; import BookList from './BookList'; function App() { let showNewOnly = false; // This flag's value is typically set based on specific logic. const filteredBooks = showNewOnly ? booksData.filter(book => book.isNewPublished) : booksData; return (); }
Show New Published Books Only
In this illustrative code snippet (non-functional but intended to
demonstrate the concept), we manipulate the BookList
component’s displayed content by passing it an array of books. Depending
on the showNewOnly
flag, this array is either all available
books or only those that are newly published, showcasing how props can
be used to dynamically adjust component output.
Managing Internal State Between Renders: useState
Building user interfaces (UI) often transcends the generation of
static HTML. Components frequently need to “remember” certain states and
respond to user interactions dynamically. For instance, when a user
clicks an “Add” button in a Product component, it’s necessary to update
the ShoppingCart component to reflect both the total price and the
updated item list.
In the previous code snippet, attempting to set the
showNewOnly
variable to true
within an event
handler does not achieve the desired effect:
function App () { let showNewOnly = false; const handleCheckboxChange = () => { showNewOnly = true; // this doesn't work }; const filteredBooks = showNewOnly ? booksData.filter(book => book.isNewPublished) : booksData; return (); };
Show New Published Books Only
This approach falls short because local variables inside a function
component do not persist between renders. When React re-renders this
component, it does so from scratch, disregarding any changes made to
local variables since these do not trigger re-renders. React remains
unaware of the need to update the component to reflect new data.
This limitation underscores the necessity for React’s
state
. Specifically, functional components leverage the
useState
hook to remember states across renders. Revisiting
the App
example, we can effectively remember the
showNewOnly
state as follows:
import React, { useState } from 'react'; import Checkbox from './Checkbox'; import BookList from './BookList'; function App () { const [showNewOnly, setShowNewOnly] = useState(false); const handleCheckboxChange = () => { setShowNewOnly(!showNewOnly); }; const filteredBooks = showNewOnly ? booksData.filter(guide => guide.isNewPublished) : booksData; return (); };
Present New Printed Books Solely
The useState
hook is a cornerstone of React’s Hooks system,
launched to allow practical elements to handle inside state. It
introduces state to practical elements, encapsulated by the next
syntax:
const [state, setState] = useState(initialState);
initialState
: This argument is the preliminary
worth of the state variable. It may be a easy worth like a quantity,
string, boolean, or a extra complicated object or array. The
initialState
is simply used throughout the first render to
initialize the state.- Return Worth:
useState
returns an array with
two components. The primary factor is the present state worth, and the
second factor is a perform that enables updating this worth. Through the use of
array destructuring, we assign names to those returned objects,
usuallystate
andsetState
, although you’ll be able to
select any legitimate variable names. state
: Represents the present worth of the
state. It is the worth that shall be used within the part’s UI and
logic.setState
: A perform to replace the state. This perform
accepts a brand new state worth or a perform that produces a brand new state based mostly
on the earlier state. When known as, it schedules an replace to the
part’s state and triggers a re-render to replicate the adjustments.
React treats state as a snapshot; updating it does not alter the
current state variable however as a substitute triggers a re-render. Throughout this
re-render, React acknowledges the up to date state, making certain the
BookList
part receives the proper information, thereby
reflecting the up to date guide checklist to the consumer. This snapshot-like
habits of state facilitates the dynamic and responsive nature of React
elements, enabling them to react intuitively to consumer interactions and
different adjustments.
Managing Aspect Results: useEffect
Earlier than diving deeper into our dialogue, it is essential to handle the
idea of unwanted effects. Negative effects are operations that work together with
the skin world from the React ecosystem. Frequent examples embrace
fetching information from a distant server or dynamically manipulating the DOM,
comparable to altering the web page title.
React is primarily involved with rendering information to the DOM and does
not inherently deal with information fetching or direct DOM manipulation. To
facilitate these unwanted effects, React supplies the useEffect
hook. This hook permits the execution of unwanted effects after React has
accomplished its rendering course of. If these unwanted effects lead to information
adjustments, React schedules a re-render to replicate these updates.
The useEffect
Hook accepts two arguments:
- A perform containing the aspect impact logic.
- An optionally available dependency array specifying when the aspect impact must be
re-invoked.
Omitting the second argument causes the aspect impact to run after
each render. Offering an empty array []
signifies that your impact
doesn’t rely on any values from props or state, thus not needing to
re-run. Together with particular values within the array means the aspect impact
solely re-executes if these values change.
When coping with asynchronous information fetching, the workflow inside
useEffect
entails initiating a community request. As soon as the information is
retrieved, it’s captured by way of the useState
hook, updating the
part’s inside state and preserving the fetched information throughout
renders. React, recognizing the state replace, undertakes one other render
cycle to include the brand new information.
This is a sensible instance about information fetching and state
administration:
import { useEffect, useState } from "react"; kind Consumer = { id: string; identify: string; }; const UserSection = ({ id }) => { const [user, setUser] = useState(); useEffect(() => { const fetchUser = async () => { const response = await fetch(`/api/customers/${id}`); const jsonData = await response.json(); setUser(jsonData); }; fetchUser(); }, tag:martinfowler.com,2024-05-21:Utilizing-markup-for-fallbacks-when-fetching-data); return
{consumer?.identify}
; };
Within the code snippet above, inside useEffect
, an
asynchronous perform fetchUser
is outlined after which
instantly invoked. This sample is important as a result of
useEffect
doesn’t instantly assist async capabilities as its
callback. The async perform is outlined to make use of await
for
the fetch operation, making certain that the code execution waits for the
response after which processes the JSON information. As soon as the information is out there,
it updates the part’s state by way of setUser
.
The dependency array tag:martinfowler.com,2024-05-21:Utilizing-markup-for-fallbacks-when-fetching-data
on the finish of the
useEffect
name ensures that the impact runs once more provided that
id
adjustments, which prevents pointless community requests on
each render and fetches new consumer information when the id
prop
updates.
This method to dealing with asynchronous information fetching inside
useEffect
is a normal apply in React improvement, providing a
structured and environment friendly option to combine async operations into the
React part lifecycle.
As well as, in sensible functions, managing totally different states
comparable to loading, error, and information presentation is crucial too (we’ll
see it the way it works within the following part). For instance, take into account
implementing standing indicators inside a Consumer part to replicate
loading, error, or information states, enhancing the consumer expertise by
offering suggestions throughout information fetching operations.
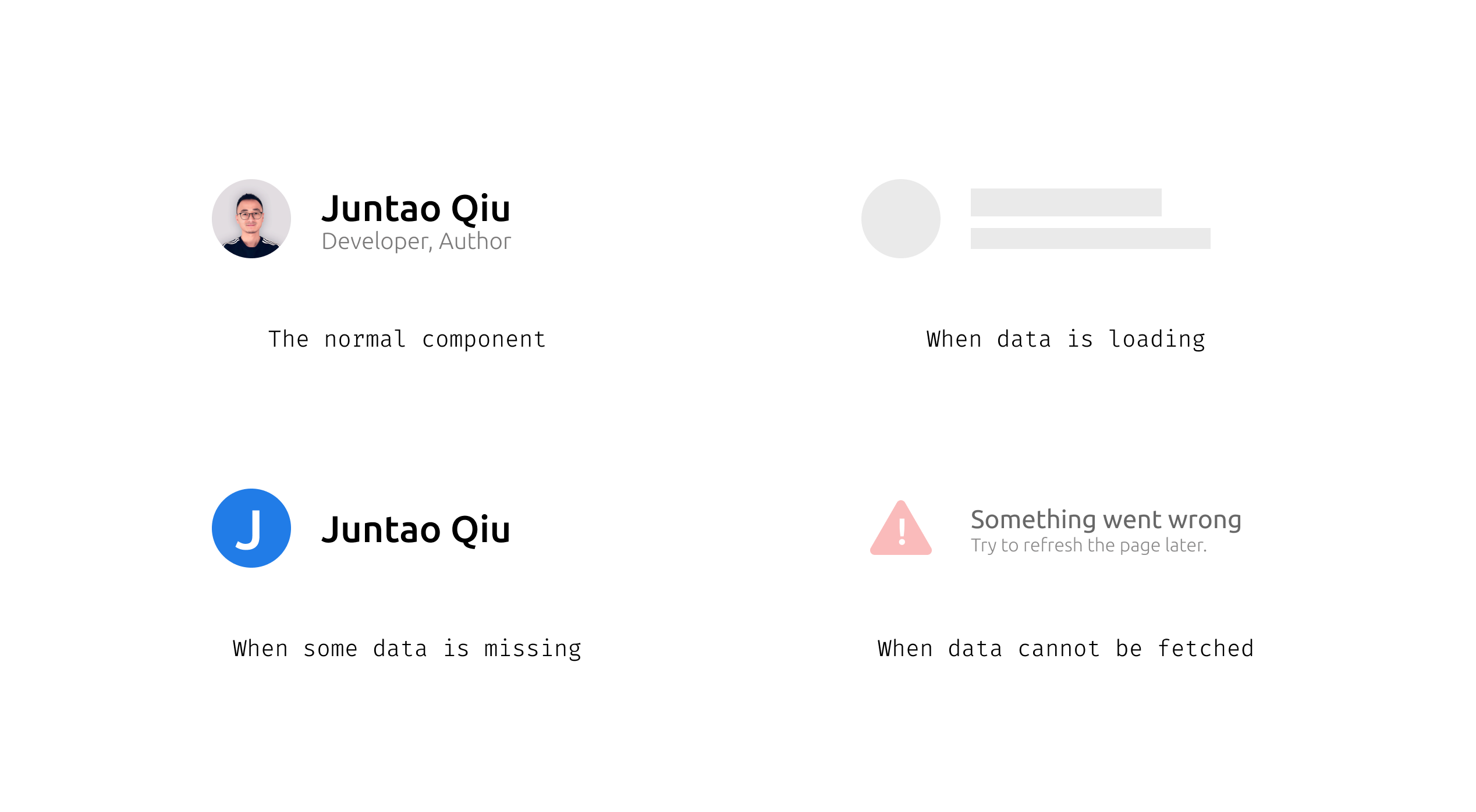
Determine 2: Totally different statuses of a
part
This overview provides only a fast glimpse into the ideas utilized
all through this text. For a deeper dive into further ideas and
patterns, I like to recommend exploring the new React
documentation or consulting different on-line assets.
With this basis, it is best to now be outfitted to affix me as we delve
into the information fetching patterns mentioned herein.
Implement the Profile part
Let’s create the Profile
part to make a request and
render the consequence. In typical React functions, this information fetching is
dealt with inside a useEffect
block. This is an instance of how
this could be applied:
import { useEffect, useState } from "react"; const Profile = ({ id }: { id: string }) => { const [user, setUser] = useState(); useEffect(() => { const fetchUser = async () => { const response = await fetch(`/api/customers/${id}`); const jsonData = await response.json(); setUser(jsonData); }; fetchUser(); }, tag:martinfowler.com,2024-05-21:Utilizing-markup-for-fallbacks-when-fetching-data); return ( ); };
This preliminary method assumes community requests full
instantaneously, which is commonly not the case. Actual-world situations require
dealing with various community circumstances, together with delays and failures. To
handle these successfully, we incorporate loading and error states into our
part. This addition permits us to offer suggestions to the consumer throughout
information fetching, comparable to displaying a loading indicator or a skeleton display screen
if the information is delayed, and dealing with errors once they happen.
Right here’s how the improved part seems with added loading and error
administration:
import { useEffect, useState } from "react"; import { get } from "../utils.ts"; import kind { Consumer } from "../varieties.ts"; const Profile = ({ id }: { id: string }) => { const [loading, setLoading] = useState(false); const [error, setError] = useState (); const [user, setUser] = useState (); useEffect(() => { const fetchUser = async () => { strive { setLoading(true); const information = await get (`/customers/${id}`); setUser(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; fetchUser(); }, tag:martinfowler.com,2024-05-21:Utilizing-markup-for-fallbacks-when-fetching-data); if (loading || !consumer) { return Loading...
; } return ( <> {consumer &&} > ); };
Now in Profile
part, we provoke states for loading,
errors, and consumer information with useState
. Utilizing
useEffect
, we fetch consumer information based mostly on id
,
toggling loading standing and dealing with errors accordingly. Upon profitable
information retrieval, we replace the consumer state, else show a loading
indicator.
The get
perform, as demonstrated beneath, simplifies
fetching information from a particular endpoint by appending the endpoint to a
predefined base URL. It checks the response’s success standing and both
returns the parsed JSON information or throws an error for unsuccessful requests,
streamlining error dealing with and information retrieval in our software. Be aware
it is pure TypeScript code and can be utilized in different non-React components of the
software.
const baseurl = "https://icodeit.com.au/api/v2"; async perform get(url: string): Promise { const response = await fetch(`${baseurl}${url}`); if (!response.okay) { throw new Error("Community response was not okay"); } return await response.json() as Promise ; }
React will attempt to render the part initially, however as the information
consumer
isn’t out there, it returns “loading…” in a
div
. Then the useEffect
is invoked, and the
request is kicked off. As soon as in some unspecified time in the future, the response returns, React
re-renders the Profile
part with consumer
fulfilled, so now you can see the consumer part with identify, avatar, and
title.
If we visualize the timeline of the above code, you will notice
the next sequence. The browser firstly downloads the HTML web page, and
then when it encounters script tags and elegance tags, it’d cease and
obtain these recordsdata, after which parse them to type the ultimate web page. Be aware
that this can be a comparatively sophisticated course of, and I’m oversimplifying
right here, however the fundamental concept of the sequence is right.
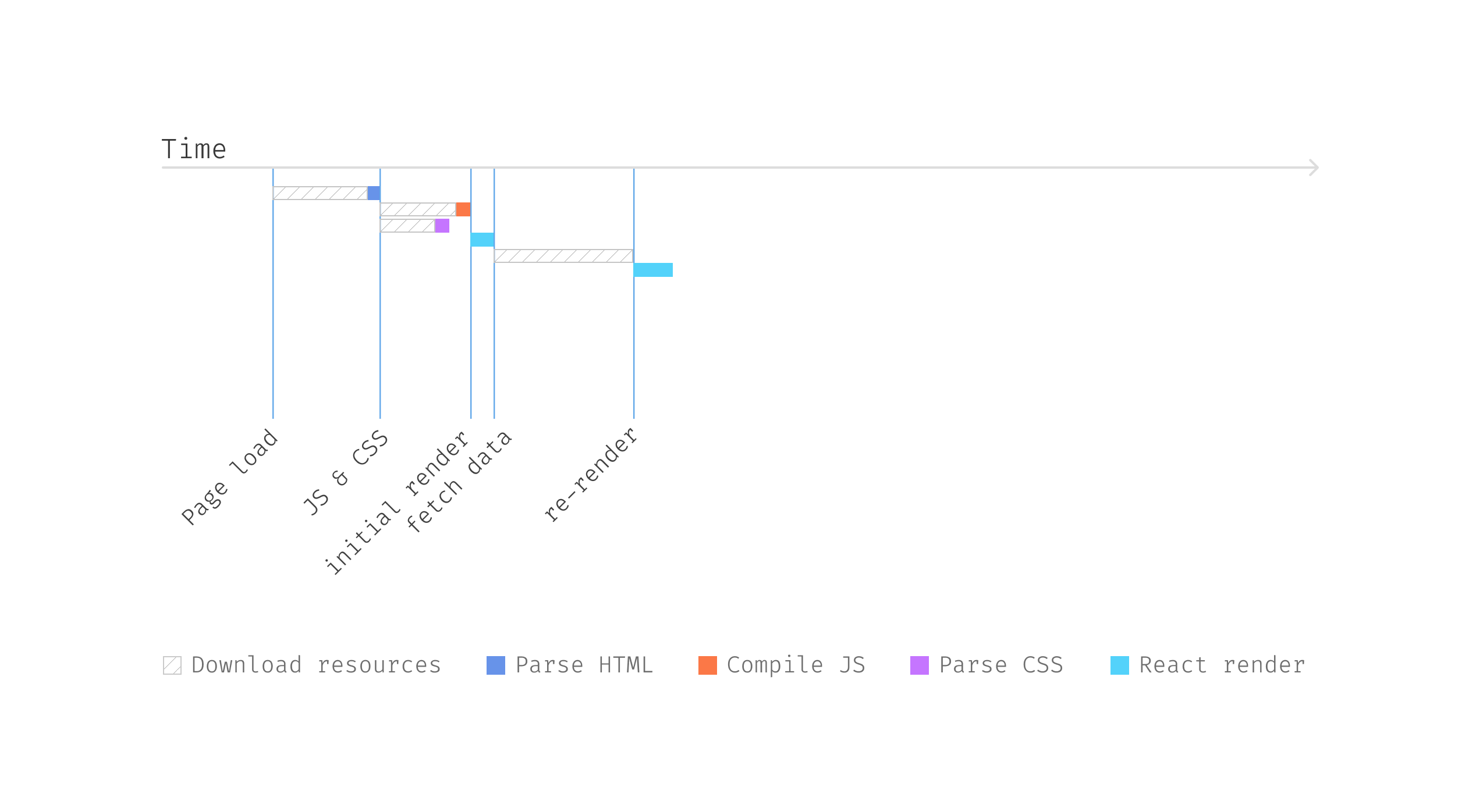
Determine 3: Fetching consumer
information
So React can begin to render solely when the JS are parsed and executed,
after which it finds the useEffect
for information fetching; it has to attend till
the information is out there for a re-render.
Now within the browser, we will see a “loading…” when the appliance
begins, after which after a couple of seconds (we will simulate such case by add
some delay within the API endpoints) the consumer temporary part reveals up when information
is loaded.
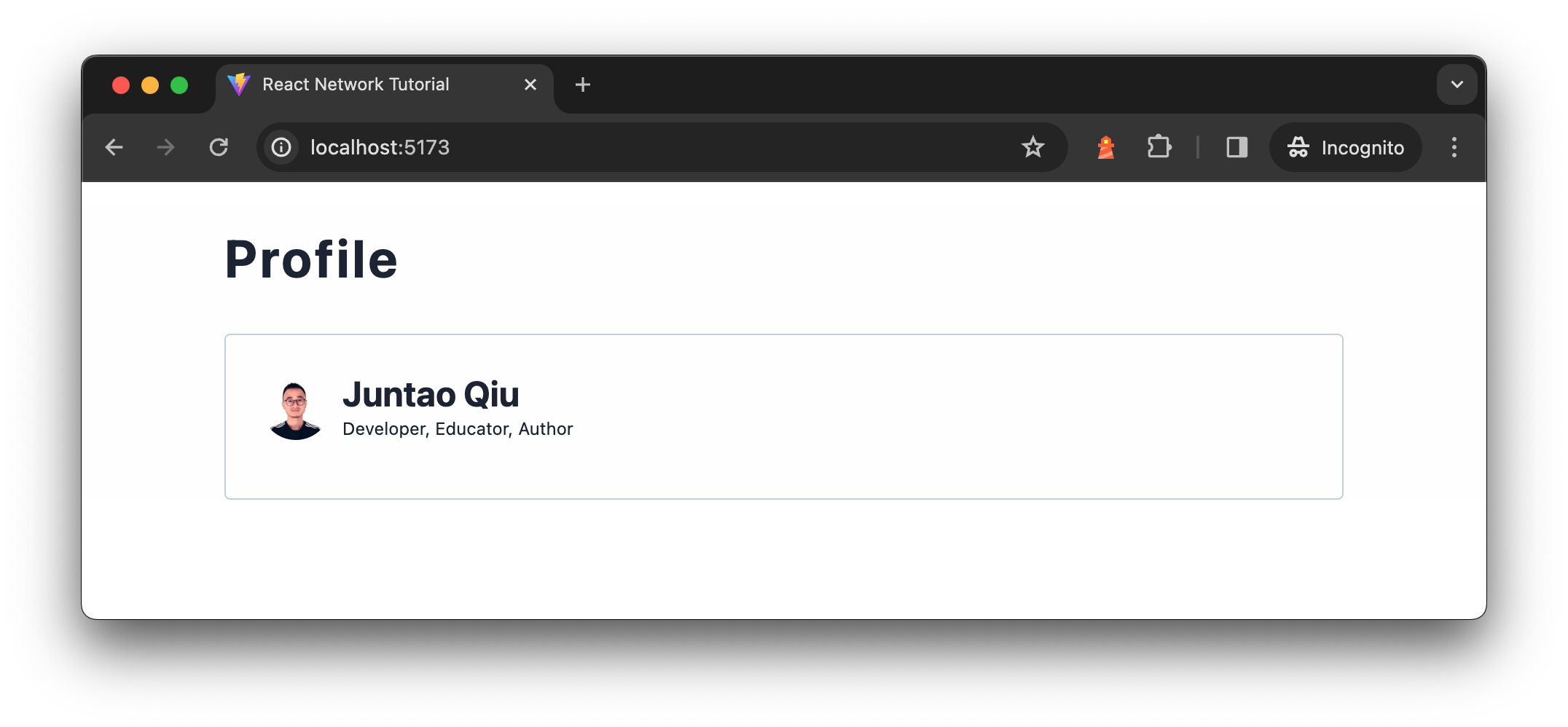
Determine 4: Consumer temporary part
This code construction (in useEffect to set off request, and replace states
like loading
and error
correspondingly) is
extensively used throughout React codebases. In functions of standard measurement, it is
frequent to search out quite a few cases of such similar data-fetching logic
dispersed all through varied elements.
Asynchronous State Handler
Wrap asynchronous queries with meta-queries for the state of the
question.
Distant calls may be gradual, and it is important to not let the UI freeze
whereas these calls are being made. Due to this fact, we deal with them asynchronously
and use indicators to indicate {that a} course of is underway, which makes the
consumer expertise higher – understanding that one thing is occurring.
Moreover, distant calls would possibly fail on account of connection points,
requiring clear communication of those failures to the consumer. Due to this fact,
it is best to encapsulate every distant name inside a handler module that
manages outcomes, progress updates, and errors. This module permits the UI
to entry metadata concerning the standing of the decision, enabling it to show
different data or choices if the anticipated outcomes fail to
materialize.
A easy implementation could possibly be a perform getAsyncStates
that
returns these metadata, it takes a URL as its parameter and returns an
object containing data important for managing asynchronous
operations. This setup permits us to appropriately reply to totally different
states of a community request, whether or not it is in progress, efficiently
resolved, or has encountered an error.
const { loading, error, information } = getAsyncStates(url); if (loading) { // Show a loading spinner } if (error) { // Show an error message } // Proceed to render utilizing the information
The idea right here is that getAsyncStates
initiates the
community request mechanically upon being known as. Nonetheless, this won’t
at all times align with the caller’s wants. To supply extra management, we will additionally
expose a fetch
perform inside the returned object, permitting
the initiation of the request at a extra applicable time, based on the
caller’s discretion. Moreover, a refetch
perform might
be offered to allow the caller to re-initiate the request as wanted,
comparable to after an error or when up to date information is required. The
fetch
and refetch
capabilities may be equivalent in
implementation, or refetch
would possibly embrace logic to examine for
cached outcomes and solely re-fetch information if vital.
const { loading, error, information, fetch, refetch } = getAsyncStates(url); const onInit = () => { fetch(); }; const onRefreshClicked = () => { refetch(); }; if (loading) { // Show a loading spinner } if (error) { // Show an error message } // Proceed to render utilizing the information
This sample supplies a flexible method to dealing with asynchronous
requests, giving builders the flexibleness to set off information fetching
explicitly and handle the UI’s response to loading, error, and success
states successfully. By decoupling the fetching logic from its initiation,
functions can adapt extra dynamically to consumer interactions and different
runtime circumstances, enhancing the consumer expertise and software
reliability.
Implementing Asynchronous State Handler in React with hooks
The sample may be applied in numerous frontend libraries. For
occasion, we might distill this method right into a customized Hook in a React
software for the Profile part:
import { useEffect, useState } from "react"; import { get } from "../utils.ts"; const useUser = (id: string) => { const [loading, setLoading] = useState(false); const [error, setError] = useState (); const [user, setUser] = useState (); useEffect(() => { const fetchUser = async () => { strive { setLoading(true); const information = await get (`/customers/${id}`); setUser(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; fetchUser(); }, tag:martinfowler.com,2024-05-21:Utilizing-markup-for-fallbacks-when-fetching-data); return { loading, error, consumer, }; };
Please be aware that within the customized Hook, we have no JSX code –
which means it’s very UI free however sharable stateful logic. And the
useUser
launch information mechanically when known as. Inside the Profile
part, leveraging the useUser
Hook simplifies its logic:
import { useUser } from './useUser.ts'; import UserBrief from './UserBrief.tsx'; const Profile = ({ id }: { id: string }) => { const { loading, error, consumer } = useUser(id); if (loading || !consumer) { returnLoading...
; } if (error) { returnOne thing went fallacious...
; } return ( <> {consumer &&} > ); };
Generalizing Parameter Utilization
In most functions, fetching various kinds of information—from consumer
particulars on a homepage to product lists in search outcomes and
suggestions beneath them—is a typical requirement. Writing separate
fetch capabilities for every kind of knowledge may be tedious and tough to
preserve. A greater method is to summary this performance right into a
generic, reusable hook that may deal with varied information varieties
effectively.
Take into account treating distant API endpoints as providers, and use a generic
useService
hook that accepts a URL as a parameter whereas managing all
the metadata related to an asynchronous request:
import { get } from "../utils.ts"; perform useService(url: string) { const [loading, setLoading] = useState (false); const [error, setError] = useState (); const [data, setData] = useState (); const fetch = async () => { strive { setLoading(true); const information = await get (url); setData(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; return { loading, error, information, fetch, }; }
This hook abstracts the information fetching course of, making it simpler to
combine into any part that should retrieve information from a distant
supply. It additionally centralizes frequent error dealing with situations, comparable to
treating particular errors in a different way:
import { useService } from './useService.ts'; const { loading, error, information: consumer, fetch: fetchUser, } = useService(`/customers/${id}`);
Through the use of useService, we will simplify how elements fetch and deal with
information, making the codebase cleaner and extra maintainable.
Variation of the sample
A variation of the useUser
can be expose the
fetchUsers
perform, and it doesn’t set off the information
fetching itself:
import { useState } from "react"; const useUser = (id: string) => { // outline the states const fetchUser = async () => { strive { setLoading(true); const information = await get(`/customers/${id}`); setUser(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; return { loading, error, consumer, fetchUser, }; };
After which on the calling website, Profile
part use
useEffect
to fetch the information and render totally different
states.
const Profile = ({ id }: { id: string }) => { const { loading, error, consumer, fetchUser } = useUser(id); useEffect(() => { fetchUser(); }, []); // render correspondingly };
The benefit of this division is the power to reuse these stateful
logics throughout totally different elements. For example, one other part
needing the identical information (a consumer API name with a consumer ID) can merely import
the useUser
Hook and make the most of its states. Totally different UI
elements would possibly select to work together with these states in varied methods,
maybe utilizing different loading indicators (a smaller spinner that
suits to the calling part) or error messages, but the basic
logic of fetching information stays constant and shared.
When to make use of it
Separating information fetching logic from UI elements can typically
introduce pointless complexity, significantly in smaller functions.
Conserving this logic built-in inside the part, much like the
css-in-js method, simplifies navigation and is simpler for some
builders to handle. In my article, Modularizing
React Purposes with Established UI Patterns, I explored
varied ranges of complexity in software constructions. For functions
which can be restricted in scope — with just some pages and a number of other information
fetching operations — it is usually sensible and in addition advisable to
preserve information fetching inside the UI elements.
Nonetheless, as your software scales and the event staff grows,
this technique might result in inefficiencies. Deep part bushes can gradual
down your software (we are going to see examples in addition to learn how to tackle
them within the following sections) and generate redundant boilerplate code.
Introducing an Asynchronous State Handler can mitigate these points by
decoupling information fetching from UI rendering, enhancing each efficiency
and maintainability.
It’s essential to steadiness simplicity with structured approaches as your
undertaking evolves. This ensures your improvement practices stay
efficient and attentive to the appliance’s wants, sustaining optimum
efficiency and developer effectivity whatever the undertaking
scale.
Implement the Associates checklist
Now let’s take a look on the second part of the Profile – the pal
checklist. We are able to create a separate part Associates
and fetch information in it
(by utilizing a useService customized hook we outlined above), and the logic is
fairly much like what we see above within the Profile
part.
const Associates = ({ id }: { id: string }) => { const { loading, error, information: associates } = useService(`/customers/${id}/associates`); // loading & error dealing with... return (); };Associates
{associates.map((consumer) => ( // render consumer checklist ))}
After which within the Profile part, we will use Associates as a daily
part, and move in id
as a prop:
const Profile = ({ id }: { id: string }) => { //... return ( <> {consumer &&} > ); };
The code works high quality, and it seems fairly clear and readable,
UserBrief
renders a consumer
object handed in, whereas
Associates
handle its personal information fetching and rendering logic
altogether. If we visualize the part tree, it might be one thing like
this:
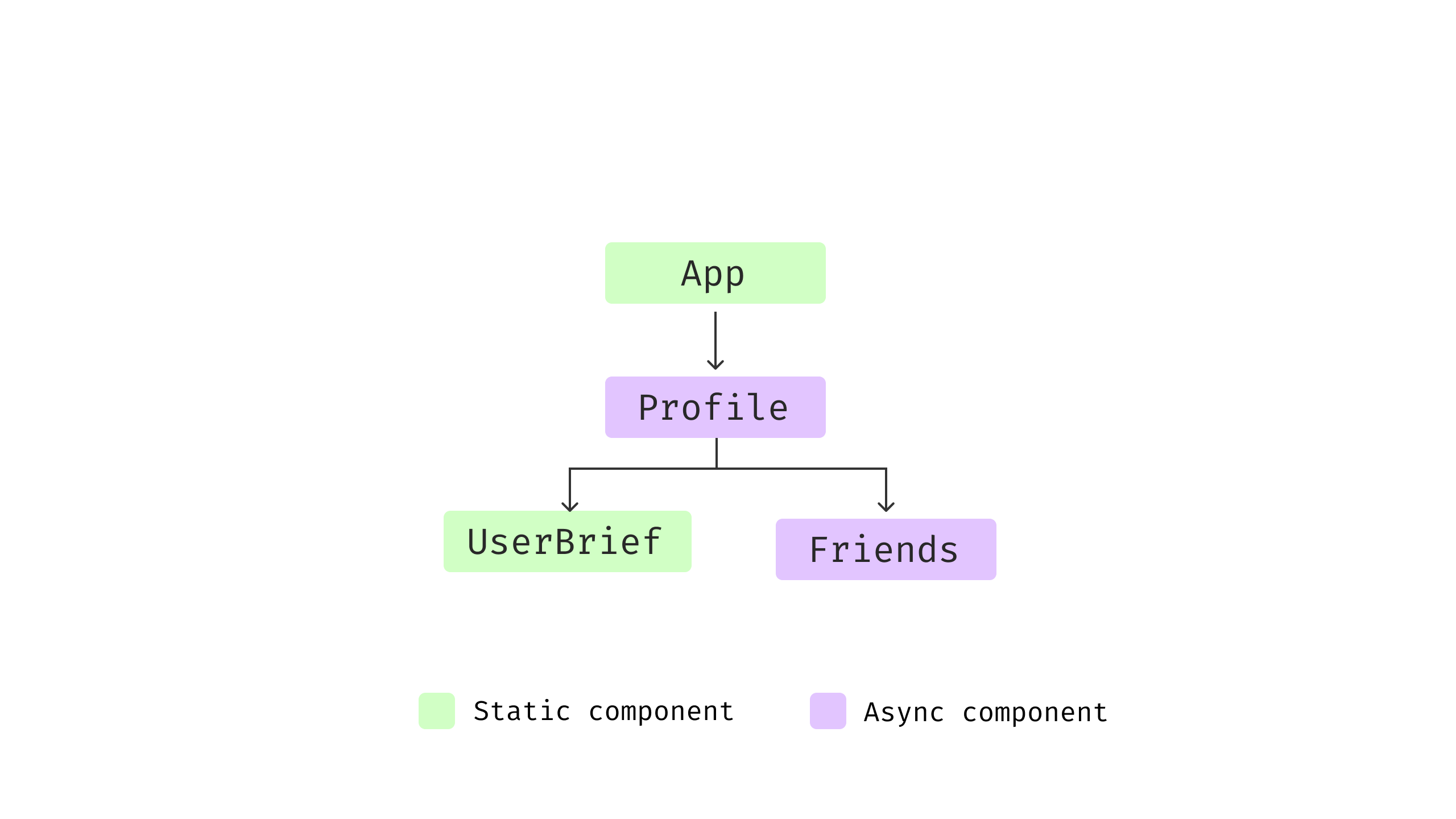
Determine 5: Element construction
Each the Profile
and Associates
have logic for
information fetching, loading checks, and error dealing with. Since there are two
separate information fetching calls, and if we have a look at the request timeline, we
will discover one thing fascinating.
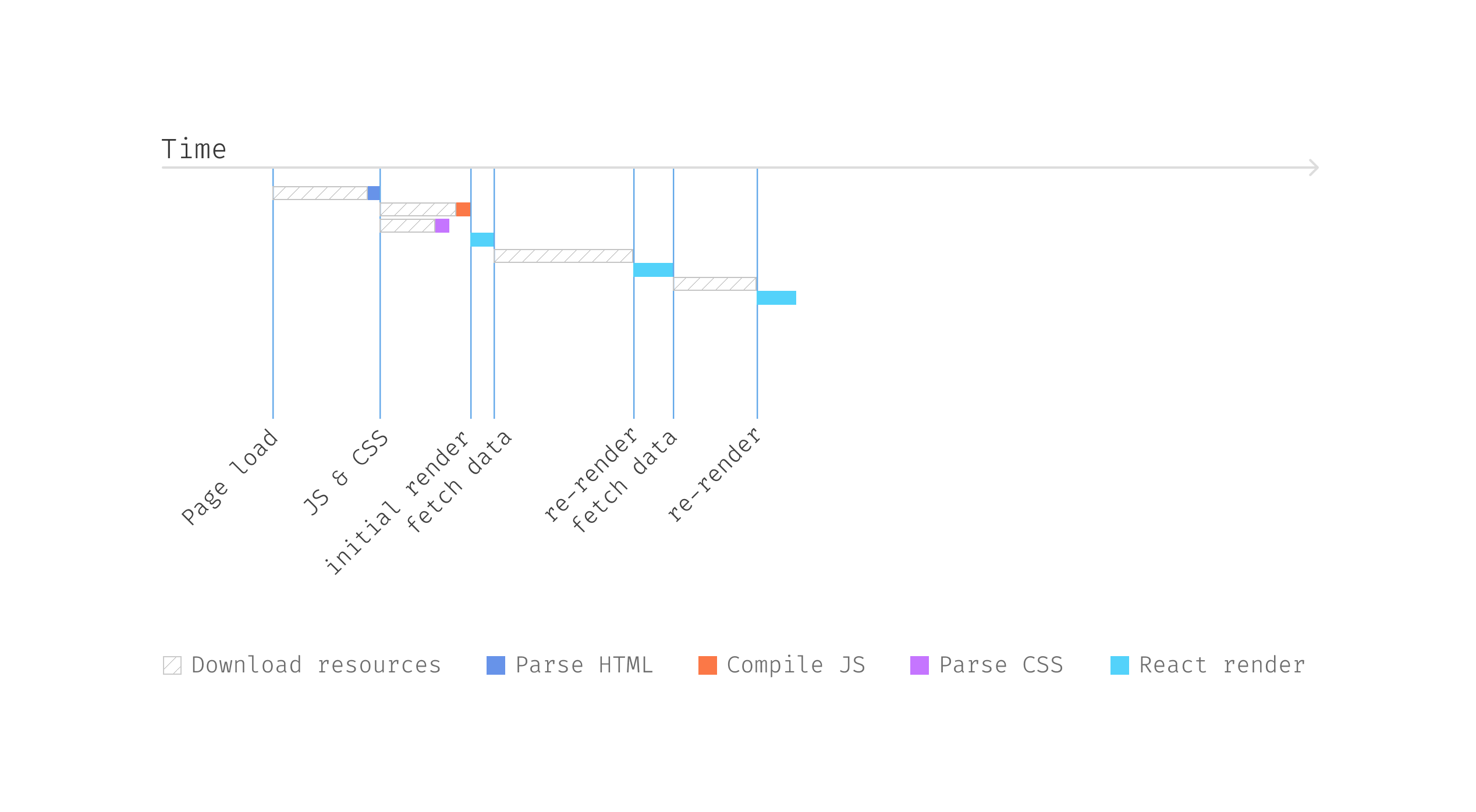
Determine 6: Request waterfall
The Associates
part will not provoke information fetching till the consumer
state is ready. That is known as the Fetch-On-Render method,
the place the preliminary rendering is paused as a result of the information is not out there,
requiring React to attend for the information to be retrieved from the server
aspect.
This ready interval is considerably inefficient, contemplating that whereas
React’s rendering course of solely takes a couple of milliseconds, information fetching can
take considerably longer, usually seconds. In consequence, the Associates
part spends most of its time idle, ready for information. This state of affairs
results in a typical problem referred to as the Request Waterfall, a frequent
incidence in frontend functions that contain a number of information fetching
operations.
Parallel Information Fetching
Run distant information fetches in parallel to attenuate wait time
Think about after we construct a bigger software {that a} part that
requires information may be deeply nested within the part tree, to make the
matter worse these elements are developed by totally different groups, it’s onerous
to see whom we’re blocking.
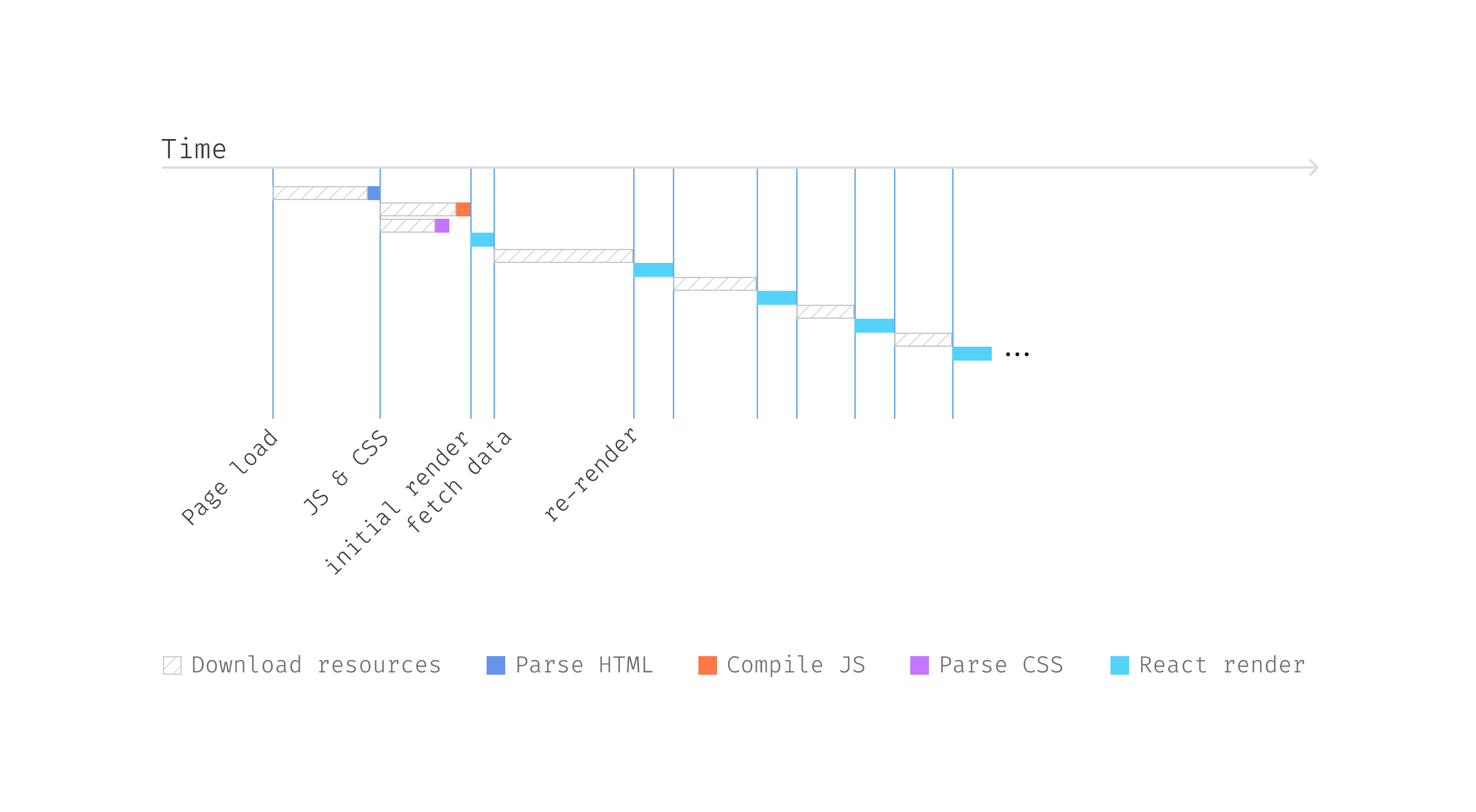
Determine 7: Request waterfall
Request Waterfalls can degrade consumer
expertise, one thing we purpose to keep away from. Analyzing the information, we see that the
consumer API and associates API are unbiased and may be fetched in parallel.
Initiating these parallel requests turns into important for software
efficiency.
One method is to centralize information fetching at the next stage, close to the
root. Early within the software’s lifecycle, we begin all information fetches
concurrently. Elements depending on this information wait just for the
slowest request, usually leading to sooner total load occasions.
We might use the Promise API Promise.all
to ship
each requests for the consumer’s fundamental data and their associates checklist.
Promise.all
is a JavaScript technique that enables for the
concurrent execution of a number of guarantees. It takes an array of guarantees
as enter and returns a single Promise that resolves when all the enter
guarantees have resolved, offering their outcomes as an array. If any of the
guarantees fail, Promise.all
instantly rejects with the
purpose of the primary promise that rejects.
For example, on the software’s root, we will outline a complete
information mannequin:
kind ProfileState = { consumer: Consumer; associates: Consumer[]; }; const getProfileData = async (id: string) => Promise.all([ get(`/users/${id}`), get (`/users/${id}/friends`), ]); const App = () => { // fetch information on the very begining of the appliance launch const onInit = () => { const [user, friends] = await getProfileData(id); } // render the sub tree correspondingly }
Implementing Parallel Information Fetching in React
Upon software launch, information fetching begins, abstracting the
fetching course of from subcomponents. For instance, in Profile part,
each UserBrief and Associates are presentational elements that react to
the handed information. This manner we might develop these part individually
(including types for various states, for instance). These presentational
elements usually are simple to check and modify as now we have separate the
information fetching and rendering.
We are able to outline a customized hook useProfileData
that facilitates
parallel fetching of knowledge associated to a consumer and their associates by utilizing
Promise.all
. This technique permits simultaneous requests, optimizing the
loading course of and structuring the information right into a predefined format identified
as ProfileData
.
Right here’s a breakdown of the hook implementation:
import { useCallback, useEffect, useState } from "react"; kind ProfileData = { consumer: Consumer; associates: Consumer[]; }; const useProfileData = (id: string) => { const [loading, setLoading] = useState(false); const [error, setError] = useState (undefined); const [profileState, setProfileState] = useState (); const fetchProfileState = useCallback(async () => { strive { setLoading(true); const [user, friends] = await Promise.all([ get (`/users/${id}`), get (`/users/${id}/friends`), ]); setProfileState({ consumer, associates }); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }, tag:martinfowler.com,2024-05-21:Utilizing-markup-for-fallbacks-when-fetching-data); return { loading, error, profileState, fetchProfileState, }; };
This hook supplies the Profile
part with the
vital information states (loading
, error
,
profileState
) together with a fetchProfileState
perform, enabling the part to provoke the fetch operation as
wanted. Be aware right here we use useCallback
hook to wrap the async
perform for information fetching. The useCallback hook in React is used to
memoize capabilities, making certain that the identical perform occasion is
maintained throughout part re-renders until its dependencies change.
Just like the useEffect, it accepts the perform and a dependency
array, the perform will solely be recreated if any of those dependencies
change, thereby avoiding unintended habits in React’s rendering
cycle.
The Profile
part makes use of this hook and controls the information fetching
timing by way of useEffect
:
const Profile = ({ id }: { id: string }) => { const { loading, error, profileState, fetchProfileState } = useProfileData(id); useEffect(() => { fetchProfileState(); }, [fetchProfileState]); if (loading) { returnLoading...
; } if (error) { returnOne thing went fallacious...
; } return ( <> {profileState && ( <>> )} > ); };
This method is often known as Fetch-Then-Render, suggesting that the purpose
is to provoke requests as early as doable throughout web page load.
Subsequently, the fetched information is utilized to drive React’s rendering of
the appliance, bypassing the necessity to handle information fetching amidst the
rendering course of. This technique simplifies the rendering course of,
making the code simpler to check and modify.
And the part construction, if visualized, can be just like the
following illustration
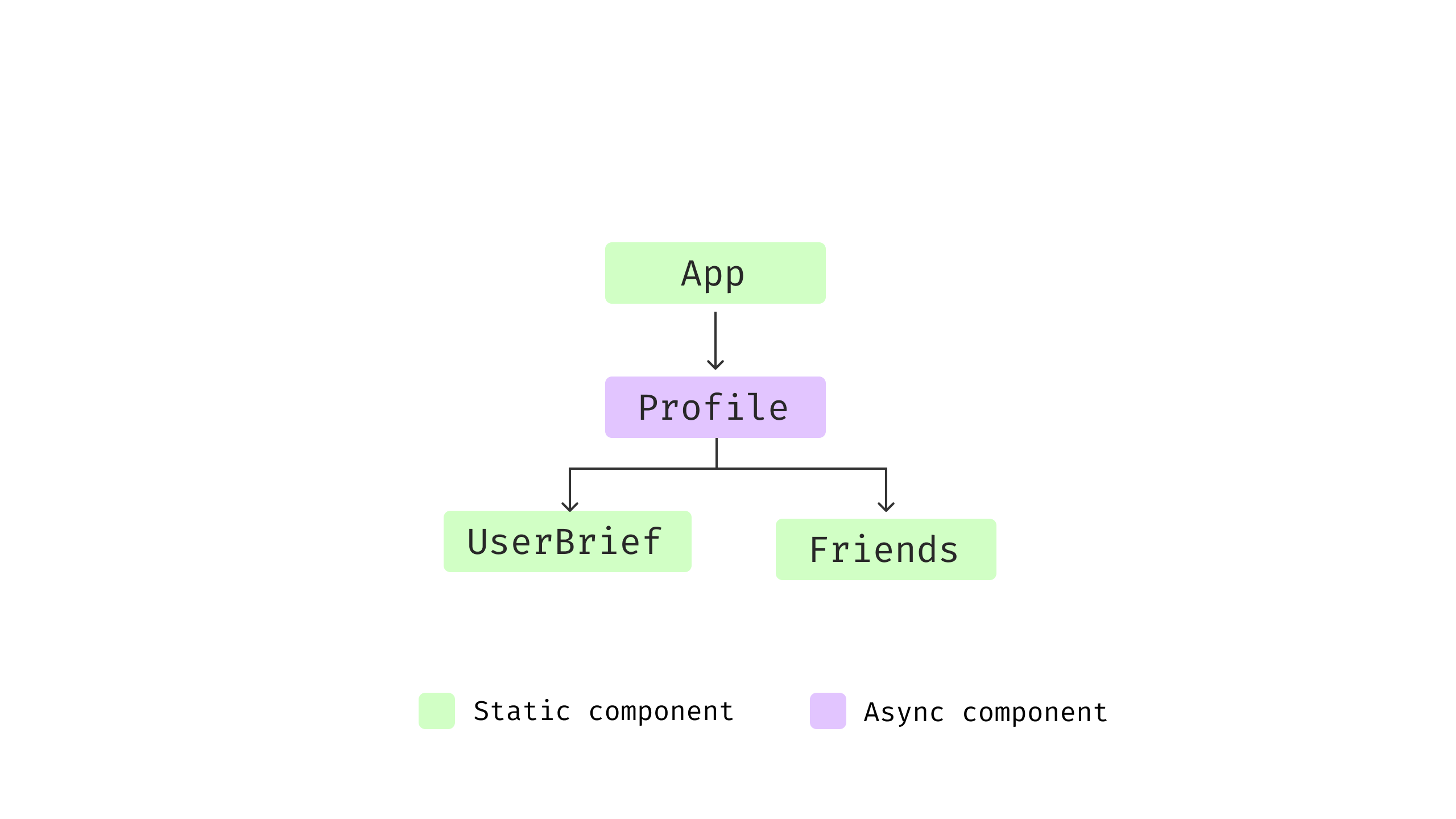
Determine 8: Element construction after refactoring
And the timeline is far shorter than the earlier one as we ship two
requests in parallel. The Associates
part can render in a couple of
milliseconds as when it begins to render, the information is already prepared and
handed in.
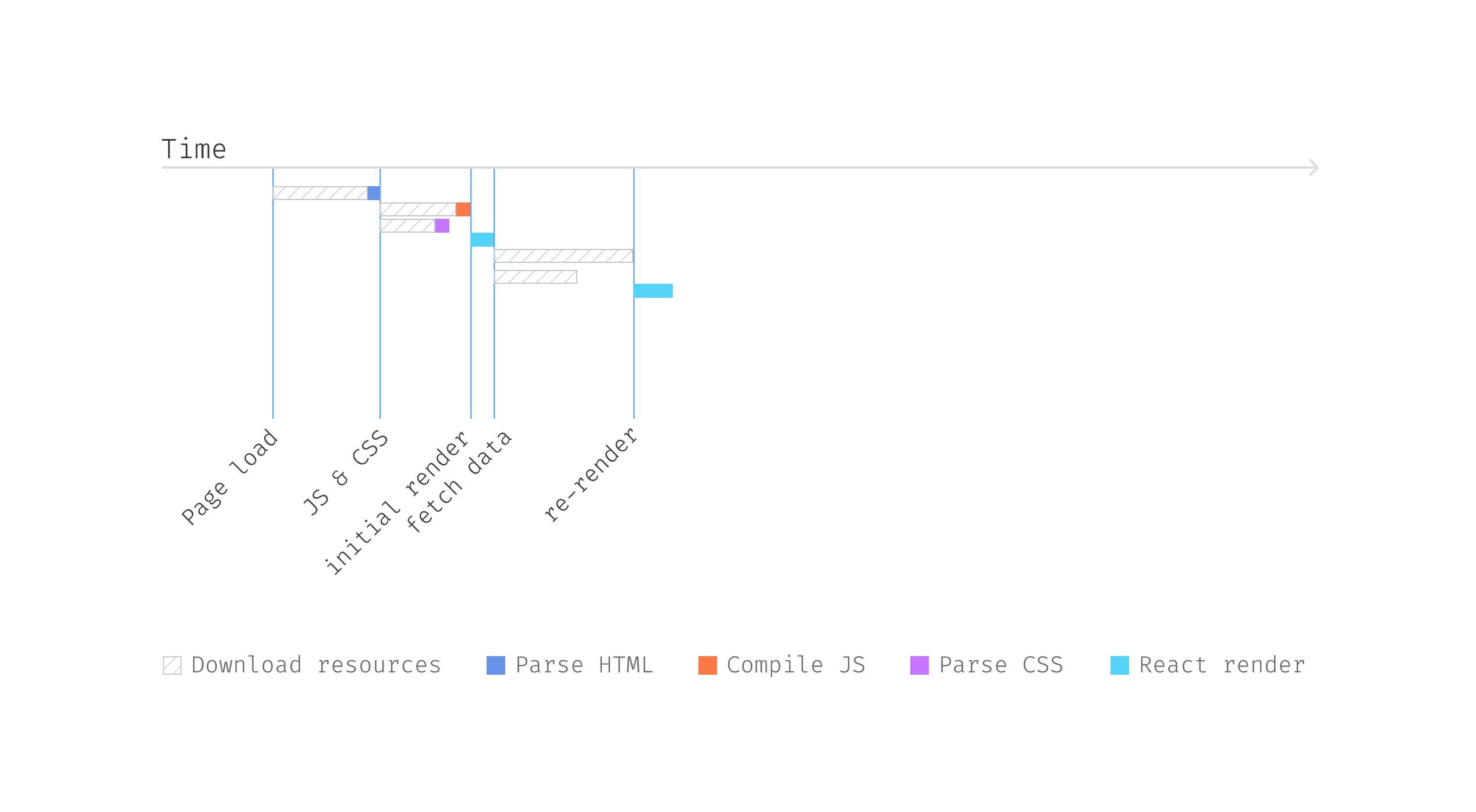
Determine 9: Parallel requests
Be aware that the longest wait time is determined by the slowest community
request, which is far sooner than the sequential ones. And if we might
ship as many of those unbiased requests on the similar time at an higher
stage of the part tree, a greater consumer expertise may be
anticipated.
As functions develop, managing an rising variety of requests at
root stage turns into difficult. That is significantly true for elements
distant from the foundation, the place passing down information turns into cumbersome. One
method is to retailer all information globally, accessible by way of capabilities (like
Redux or the React Context API), avoiding deep prop drilling.
When to make use of it
Operating queries in parallel is beneficial every time such queries could also be
gradual and do not considerably intervene with every others’ efficiency.
That is normally the case with distant queries. Even when the distant
machine’s I/O and computation is quick, there’s at all times potential latency
points within the distant calls. The principle drawback for parallel queries
is setting them up with some form of asynchronous mechanism, which can be
tough in some language environments.
The principle purpose to not use parallel information fetching is after we do not
know what information must be fetched till we have already fetched some
information. Sure situations require sequential information fetching on account of
dependencies between requests. For example, take into account a state of affairs on a
Profile
web page the place producing a personalised advice feed
is determined by first buying the consumer’s pursuits from a consumer API.
This is an instance response from the consumer API that features
pursuits:
{ "id": "u1", "identify": "Juntao Qiu", "bio": "Developer, Educator, Writer", "pursuits": [ "Technology", "Outdoors", "Travel" ] }
In such instances, the advice feed can solely be fetched after
receiving the consumer’s pursuits from the preliminary API name. This
sequential dependency prevents us from using parallel fetching, as
the second request depends on information obtained from the primary.
Given these constraints, it turns into vital to debate different
methods in asynchronous information administration. One such technique is
Fallback Markup. This method permits builders to specify what
information is required and the way it must be fetched in a approach that clearly
defines dependencies, making it simpler to handle complicated information
relationships in an software.
One other instance of when arallel Information Fetching isn’t relevant is
that in situations involving consumer interactions that require real-time
information validation.
Take into account the case of an inventory the place every merchandise has an “Approve” context
menu. When a consumer clicks on the “Approve” choice for an merchandise, a dropdown
menu seems providing decisions to both “Approve” or “Reject.” If this
merchandise’s approval standing could possibly be modified by one other admin concurrently,
then the menu choices should replicate probably the most present state to keep away from
conflicting actions.
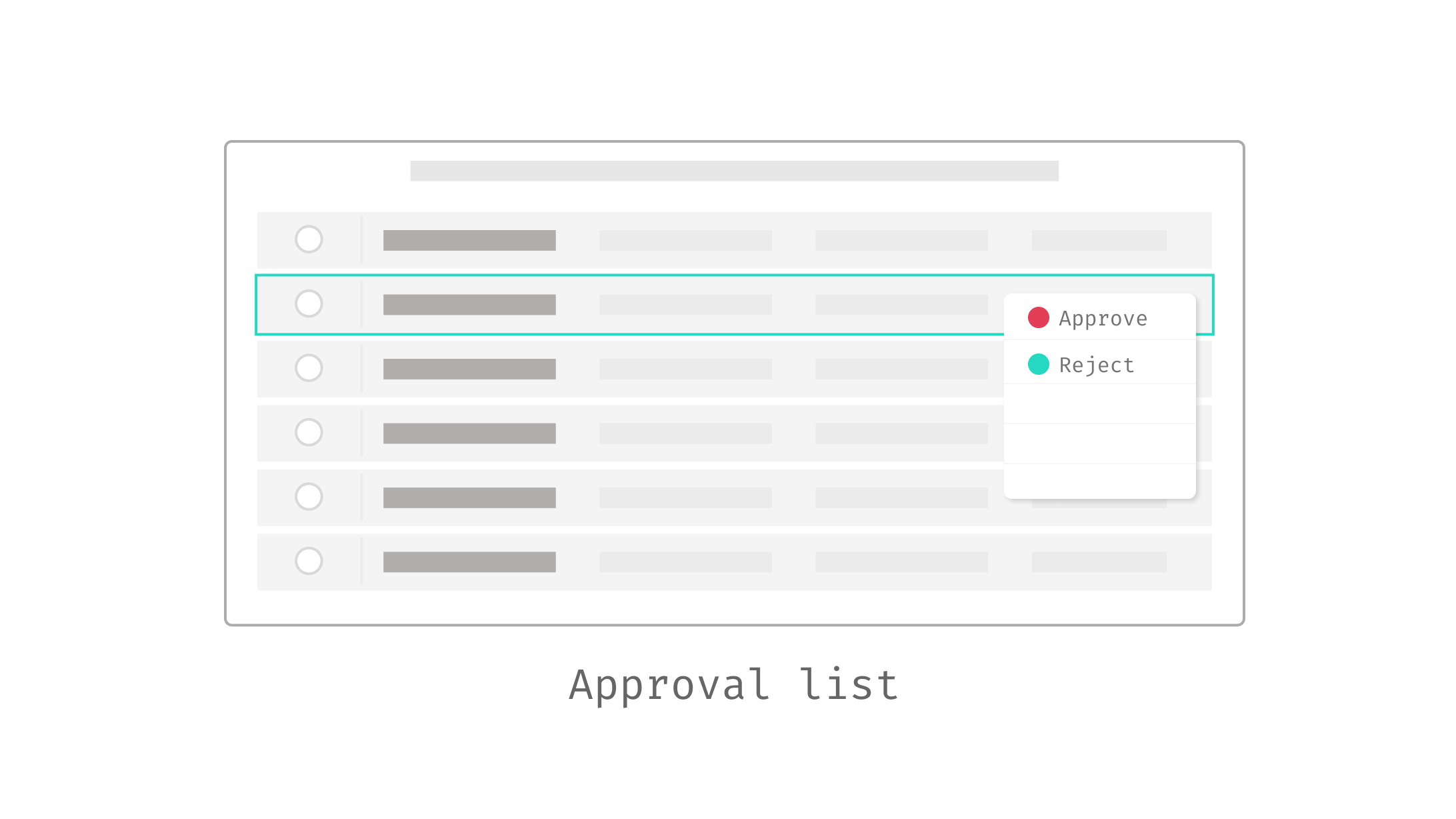
Determine 10: The approval checklist that require in-time
states
To deal with this, a service name is initiated every time the context
menu is activated. This service fetches the newest standing of the merchandise,
making certain that the dropdown is constructed with probably the most correct and
present choices out there at that second. In consequence, these requests
can’t be made in parallel with different data-fetching actions because the
dropdown’s contents rely completely on the real-time standing fetched from
the server.
Fallback Markup
Specify fallback shows within the web page markup
This sample leverages abstractions offered by frameworks or libraries
to deal with the information retrieval course of, together with managing states like
loading, success, and error, behind the scenes. It permits builders to
concentrate on the construction and presentation of knowledge of their functions,
selling cleaner and extra maintainable code.
Let’s take one other have a look at the Associates
part within the above
part. It has to take care of three totally different states and register the
callback in useEffect
, setting the flag accurately on the proper time,
prepare the totally different UI for various states:
const Associates = ({ id }: { id: string }) => { //... const { loading, error, information: associates, fetch: fetchFriends, } = useService(`/customers/${id}/associates`); useEffect(() => { fetchFriends(); }, []); if (loading) { // present loading indicator } if (error) { // present error message part } // present the acutal pal checklist };
You’ll discover that inside a part now we have to cope with
totally different states, even we extract customized Hook to scale back the noise in a
part, we nonetheless have to pay good consideration to dealing with
loading
and error
inside a part. These
boilerplate code may be cumbersome and distracting, usually cluttering the
readability of our codebase.
If we consider declarative API, like how we construct our UI with JSX, the
code may be written within the following method that lets you concentrate on
what the part is doing – not learn how to do it:
}> }>
Within the above code snippet, the intention is easy and clear: when an
error happens, ErrorMessage
is displayed. Whereas the operation is in
progress, Loading is proven. As soon as the operation completes with out errors,
the Associates part is rendered.
And the code snippet above is fairly similiar to what already be
applied in a couple of libraries (together with React and Vue.js). For instance,
the brand new Suspense
in React permits builders to extra successfully handle
asynchronous operations inside their elements, enhancing the dealing with of
loading states, error states, and the orchestration of concurrent
duties.
Implementing Fallback Markup in React with Suspense
Suspense
in React is a mechanism for effectively dealing with
asynchronous operations, comparable to information fetching or useful resource loading, in a
declarative method. By wrapping elements in a Suspense
boundary,
builders can specify fallback content material to show whereas ready for the
part’s information dependencies to be fulfilled, streamlining the consumer
expertise throughout loading states.
Whereas with the Suspense API, within the Associates
you describe what you
wish to get after which render:
import useSWR from "swr"; import { get } from "../utils.ts"; perform Associates({ id }: { id: string }) { const { information: customers } = useSWR("/api/profile", () => get(`/customers/${id}/associates`), { suspense: true, }); return ( ); }Associates
{associates.map((consumer) => (
))}
And declaratively whenever you use the Associates
, you utilize
Suspense
boundary to wrap across the Associates
part:
}>
Suspense
manages the asynchronous loading of the
Associates
part, displaying a FriendsSkeleton
placeholder till the part’s information dependencies are
resolved. This setup ensures that the consumer interface stays responsive
and informative throughout information fetching, enhancing the general consumer
expertise.
Use the sample in Vue.js
It is value noting that Vue.js can also be exploring an identical
experimental sample, the place you’ll be able to make use of Fallback Markup utilizing:
Loading...
Upon the primary render,
makes an attempt to render
its default content material behind the scenes. Ought to it encounter any
asynchronous dependencies throughout this section, it transitions right into a
pending state, the place the fallback content material is displayed as a substitute. As soon as all
the asynchronous dependencies are efficiently loaded,
strikes to a resolved state, and the content material
initially supposed for show (the default slot content material) is
rendered.
Deciding Placement for the Loading Element
You could marvel the place to put the FriendsSkeleton
part and who ought to handle it. Usually, with out utilizing Fallback
Markup, this choice is easy and dealt with instantly inside the
part that manages the information fetching:
const Associates = ({ id }: { id: string }) => { // Information fetching logic right here... if (loading) { // Show loading indicator } if (error) { // Show error message part } // Render the precise pal checklist };
On this setup, the logic for displaying loading indicators or error
messages is of course located inside the Associates
part. Nonetheless,
adopting Fallback Markup shifts this duty to the
part’s shopper:
}>
In real-world functions, the optimum method to dealing with loading
experiences relies upon considerably on the specified consumer interplay and
the construction of the appliance. For example, a hierarchical loading
method the place a father or mother part ceases to indicate a loading indicator
whereas its kids elements proceed can disrupt the consumer expertise.
Thus, it is essential to fastidiously take into account at what stage inside the
part hierarchy the loading indicators or skeleton placeholders
must be displayed.
Consider Associates
and FriendsSkeleton
as two
distinct part states—one representing the presence of knowledge, and the
different, the absence. This idea is considerably analogous to utilizing a Speical Case sample in object-oriented
programming, the place FriendsSkeleton
serves because the ‘null’
state dealing with for the Associates
part.
The hot button is to find out the granularity with which you wish to
show loading indicators and to take care of consistency in these
choices throughout your software. Doing so helps obtain a smoother and
extra predictable consumer expertise.
When to make use of it
Utilizing Fallback Markup in your UI simplifies code by enhancing its readability
and maintainability. This sample is especially efficient when using
normal elements for varied states comparable to loading, errors, skeletons, and
empty views throughout your software. It reduces redundancy and cleans up
boilerplate code, permitting elements to focus solely on rendering and
performance.
Fallback Markup, comparable to React’s Suspense, standardizes the dealing with of
asynchronous loading, making certain a constant consumer expertise. It additionally improves
software efficiency by optimizing useful resource loading and rendering, which is
particularly helpful in complicated functions with deep part bushes.
Nonetheless, the effectiveness of Fallback Markup is determined by the capabilities of
the framework you might be utilizing. For instance, React’s implementation of Suspense for
information fetching nonetheless requires third-party libraries, and Vue’s assist for
related options is experimental. Furthermore, whereas Fallback Markup can cut back
complexity in managing state throughout elements, it could introduce overhead in
less complicated functions the place managing state instantly inside elements might
suffice. Moreover, this sample might restrict detailed management over loading and
error states—conditions the place totally different error varieties want distinct dealing with would possibly
not be as simply managed with a generic fallback method.
Introducing UserDetailCard part
Let’s say we want a function that when customers hover on high of a Pal
,
we present a popup to allow them to see extra particulars about that consumer.
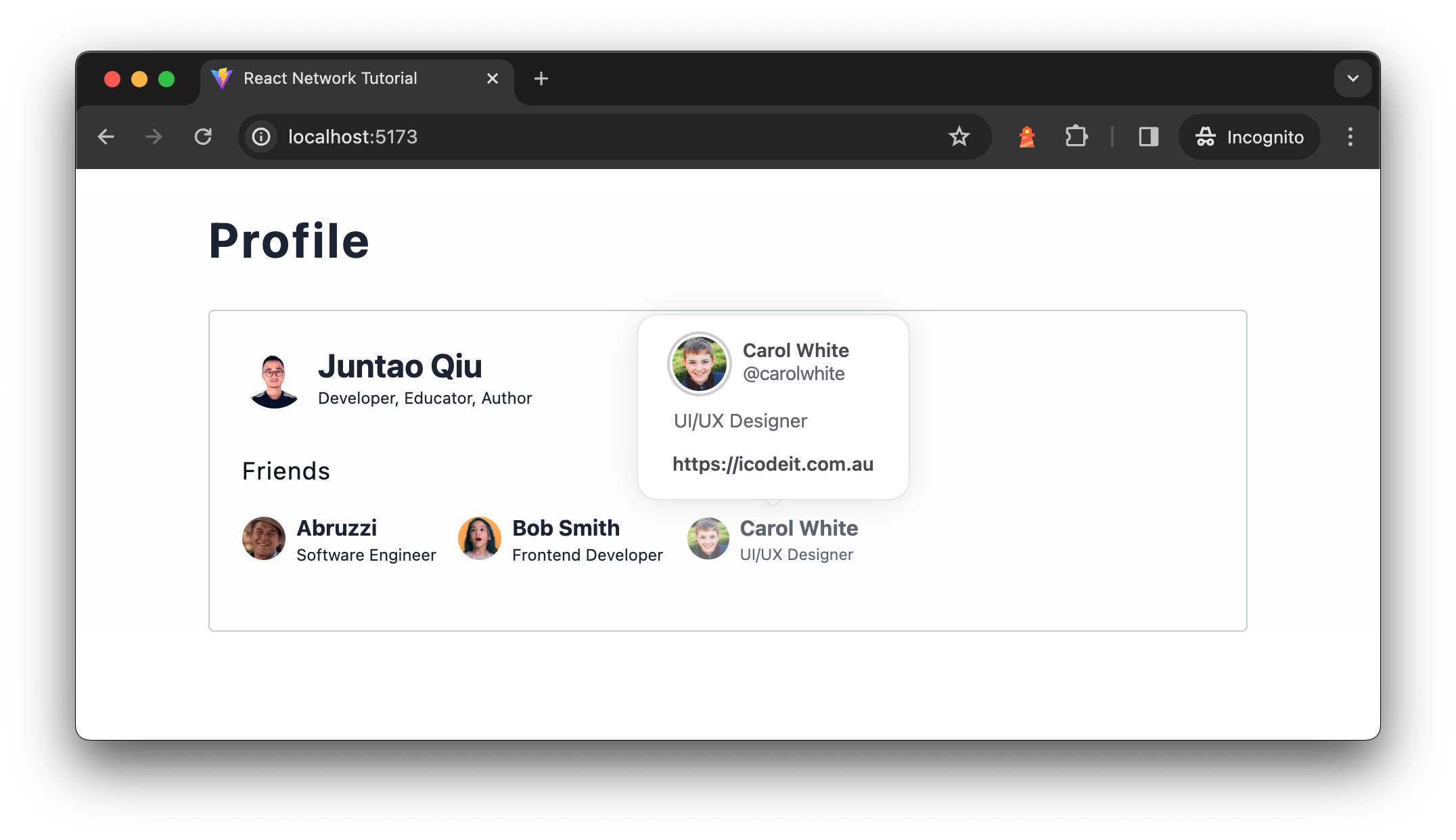
Determine 11: Exhibiting consumer element
card part when hover
When the popup reveals up, we have to ship one other service name to get
the consumer particulars (like their homepage and variety of connections, and so forth.). We
might want to replace the Pal
part ((the one we use to
render every merchandise within the Associates checklist) ) to one thing just like the
following.
import { Popover, PopoverContent, PopoverTrigger } from "@nextui-org/react"; import { UserBrief } from "./consumer.tsx"; import UserDetailCard from "./user-detail-card.tsx"; export const Pal = ({ consumer }: { consumer: Consumer }) => { return (); };
The UserDetailCard
, is fairly much like the
Profile
part, it sends a request to load information after which
renders the consequence as soon as it will get the response.
export perform UserDetailCard({ id }: { id: string }) { const { loading, error, element } = useUserDetail(id); if (loading || !element) { returnLoading...
; } return ({/* render the consumer element*/}
); }
We’re utilizing Popover
and the supporting elements from
nextui
, which supplies a whole lot of lovely and out-of-box
elements for constructing trendy UI. The one drawback right here, nevertheless, is that
the package deal itself is comparatively large, additionally not everybody makes use of the function
(hover and present particulars), so loading that additional massive package deal for everybody
isn’t splendid – it might be higher to load the UserDetailCard
on demand – every time it’s required.
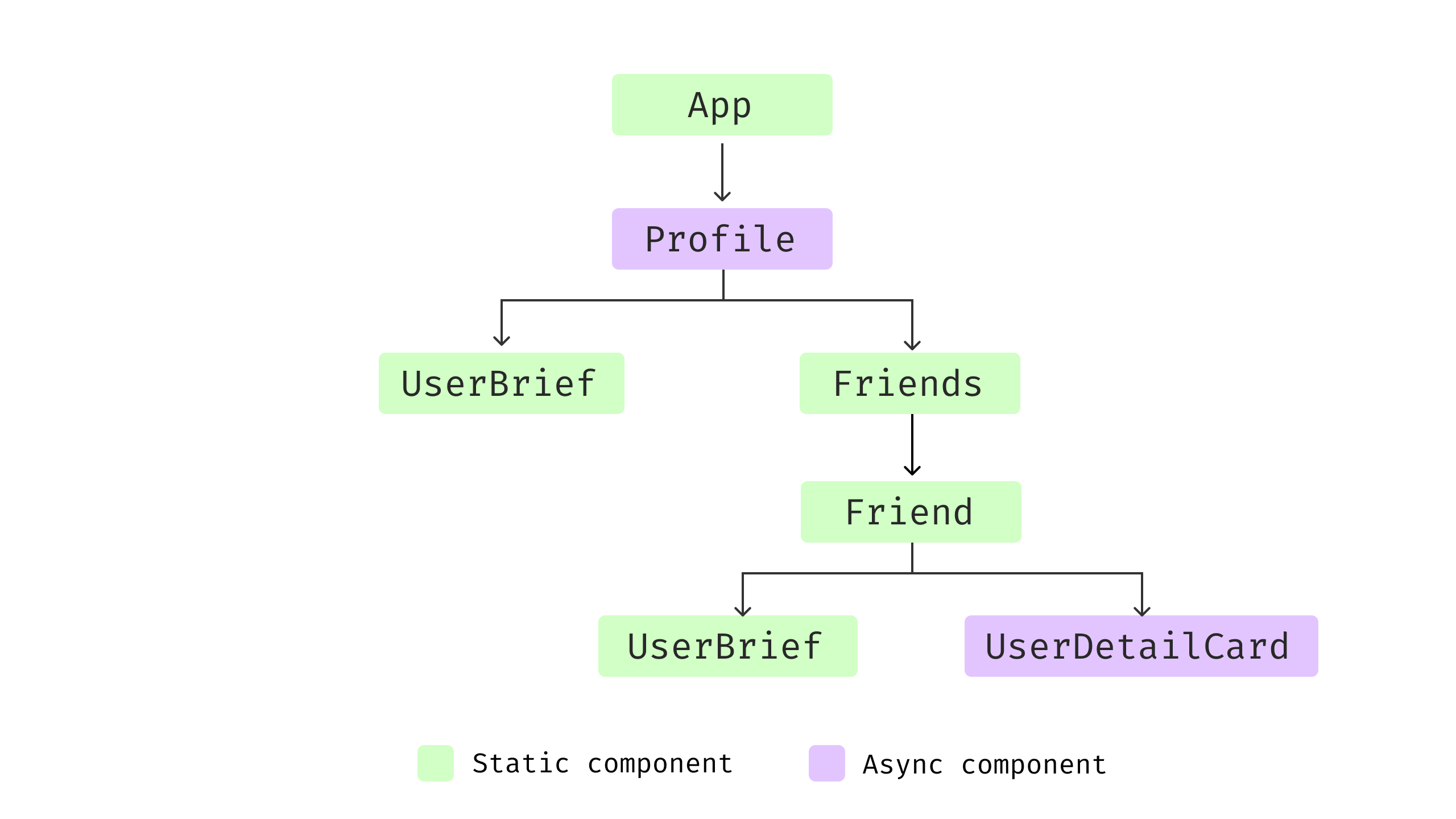
Determine 12: Element construction with
UserDetailCard
Code Splitting
Divide code into separate modules and dynamically load them as
wanted.
Code Splitting addresses the difficulty of enormous bundle sizes in internet
functions by dividing the bundle into smaller chunks which can be loaded as
wanted, relatively than abruptly. This improves preliminary load time and
efficiency, particularly vital for giant functions or these with
many routes.
This optimization is usually carried out at construct time, the place complicated
or sizable modules are segregated into distinct bundles. These are then
dynamically loaded, both in response to consumer interactions or
preemptively, in a way that doesn’t hinder the important rendering path
of the appliance.
Leveraging the Dynamic Import Operator
The dynamic import operator in JavaScript streamlines the method of
loading modules. Although it could resemble a perform name in your code,
comparable to import("./user-detail-card.tsx")
, it is vital to
acknowledge that import
is definitely a key phrase, not a
perform. This operator permits the asynchronous and dynamic loading of
JavaScript modules.
With dynamic import, you’ll be able to load a module on demand. For instance, we
solely load a module when a button is clicked:
button.addEventListener("click on", (e) => { import("/modules/some-useful-module.js") .then((module) => { module.doSomethingInteresting(); }) .catch(error => { console.error("Didn't load the module:", error); }); });
The module isn’t loaded throughout the preliminary web page load. As an alternative, the
import()
name is positioned inside an occasion listener so it solely
be loaded when, and if, the consumer interacts with that button.
You should use dynamic import operator in React and libraries like
Vue.js. React simplifies the code splitting and lazy load by the
React.lazy
and Suspense
APIs. By wrapping the
import assertion with React.lazy
, and subsequently wrapping
the part, as an example, UserDetailCard
, with
Suspense
, React defers the part rendering till the
required module is loaded. Throughout this loading section, a fallback UI is
offered, seamlessly transitioning to the precise part upon load
completion.
import React, { Suspense } from "react"; import { Popover, PopoverContent, PopoverTrigger } from "@nextui-org/react"; import { UserBrief } from "./consumer.tsx"; const UserDetailCard = React.lazy(() => import("./user-detail-card.tsx")); export const Pal = ({ consumer }: { consumer: Consumer }) => { return (Loading...
This snippet defines a Pal
part displaying consumer
particulars inside a popover from Subsequent UI, which seems upon interplay.
It leverages React.lazy
for code splitting, loading the
UserDetailCard
part solely when wanted. This
lazy-loading, mixed with Suspense
, enhances efficiency
by splitting the bundle and displaying a fallback throughout the load.
If we visualize the above code, it renders within the following
sequence.
Be aware that when the consumer hovers and we obtain
the JavaScript bundle, there shall be some additional time for the browser to
parse the JavaScript. As soon as that a part of the work is finished, we will get the
consumer particulars by calling /customers/
API.
Ultimately, we will use that information to render the content material of the popup
UserDetailCard
.
Prefetching
Prefetch information earlier than it could be wanted to scale back latency whether it is.
Prefetching entails loading assets or information forward of their precise
want, aiming to lower wait occasions throughout subsequent operations. This
approach is especially helpful in situations the place consumer actions can
be predicted, comparable to navigating to a special web page or displaying a modal
dialog that requires distant information.
In apply, prefetching may be
applied utilizing the native HTML tag with a
rel="preload"
attribute, or programmatically by way of the
fetch
API to load information or assets prematurely. For information that
is predetermined, the only method is to make use of the
tag inside the HTML
:
With this setup, the requests for bootstrap.js
and consumer API are despatched
as quickly because the HTML is parsed, considerably sooner than when different
scripts are processed. The browser will then cache the information, making certain it
is prepared when your software initializes.
Nonetheless, it is usually not doable to know the exact URLs forward of
time, requiring a extra dynamic method to prefetching. That is usually
managed programmatically, usually by occasion handlers that set off
prefetching based mostly on consumer interactions or different circumstances.
For instance, attaching a mouseover
occasion listener to a button can
set off the prefetching of knowledge. This technique permits the information to be fetched
and saved, maybe in an area state or cache, prepared for speedy use
when the precise part or content material requiring the information is interacted with
or rendered. This proactive loading minimizes latency and enhances the
consumer expertise by having information prepared forward of time.
doc.getElementById('button').addEventListener('mouseover', () => { fetch(`/consumer/${consumer.id}/particulars`) .then(response => response.json()) .then(information => { sessionStorage.setItem('userDetails', JSON.stringify(information)); }) .catch(error => console.error(error)); });
And within the place that wants the information to render, it reads from
sessionStorage
when out there, in any other case displaying a loading indicator.
Usually the consumer experiense can be a lot sooner.
Implementing Prefetching in React
For instance, we will use preload
from the
swr
package deal (the perform identify is a bit deceptive, nevertheless it
is performing a prefetch right here), after which register an
onMouseEnter
occasion to the set off part of
Popover
,
import { preload } from "swr"; import { getUserDetail } from "../api.ts"; const UserDetailCard = React.lazy(() => import("./user-detail-card.tsx")); export const Pal = ({ consumer }: { consumer: Consumer }) => { const handleMouseEnter = () => { preload(`/consumer/${consumer.id}/particulars`, () => getUserDetail(consumer.id)); }; return (); }; Loading...}>
That approach, the popup itself can have a lot much less time to render, which
brings a greater consumer expertise.
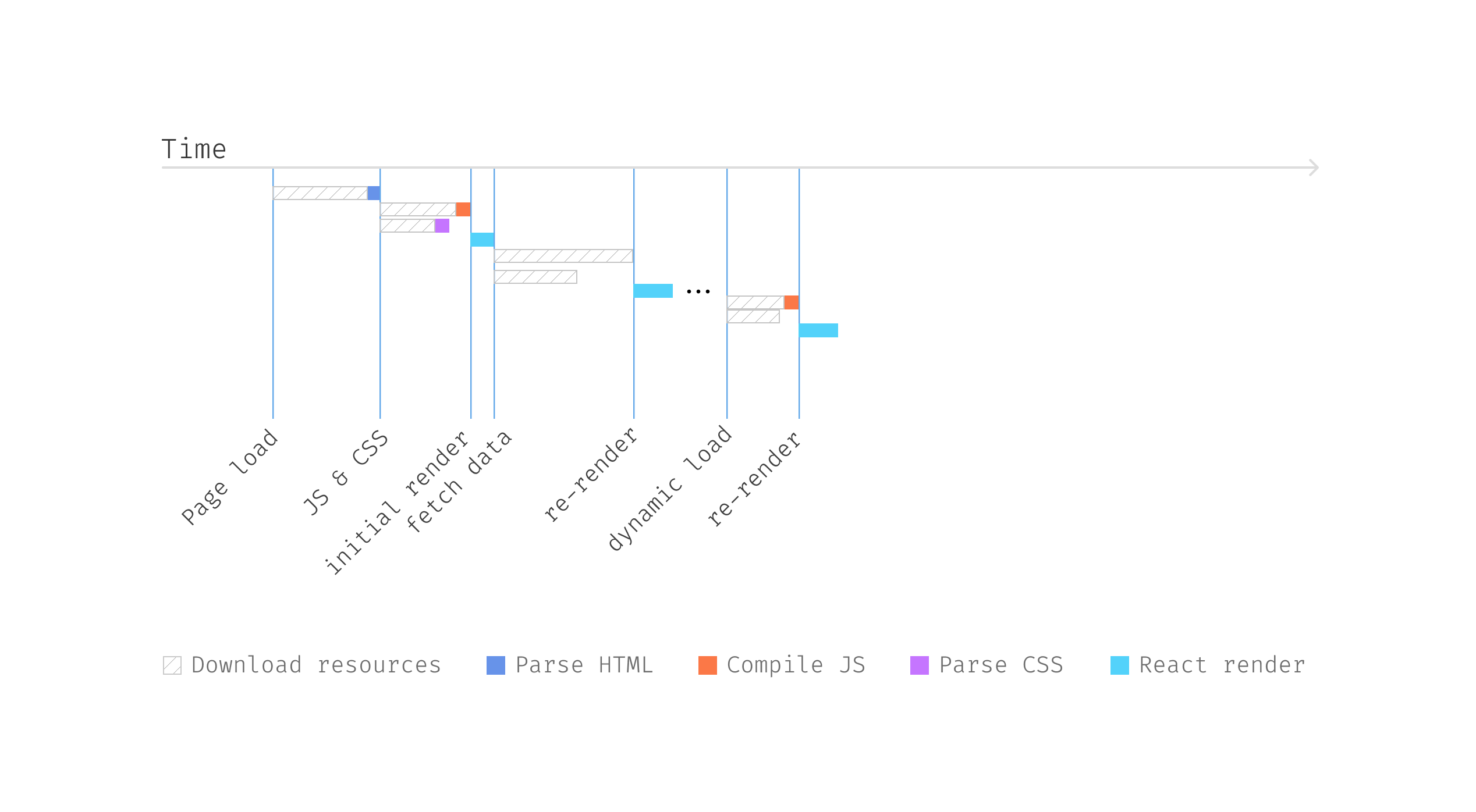
Determine 14: Dynamic load with prefetch
in parallel
So when a consumer hovers on a Pal
, we obtain the
corresponding JavaScript bundle in addition to obtain the information wanted to
render the UserDetailCard, and by the point UserDetailCard
renders, it sees the present information and renders instantly.
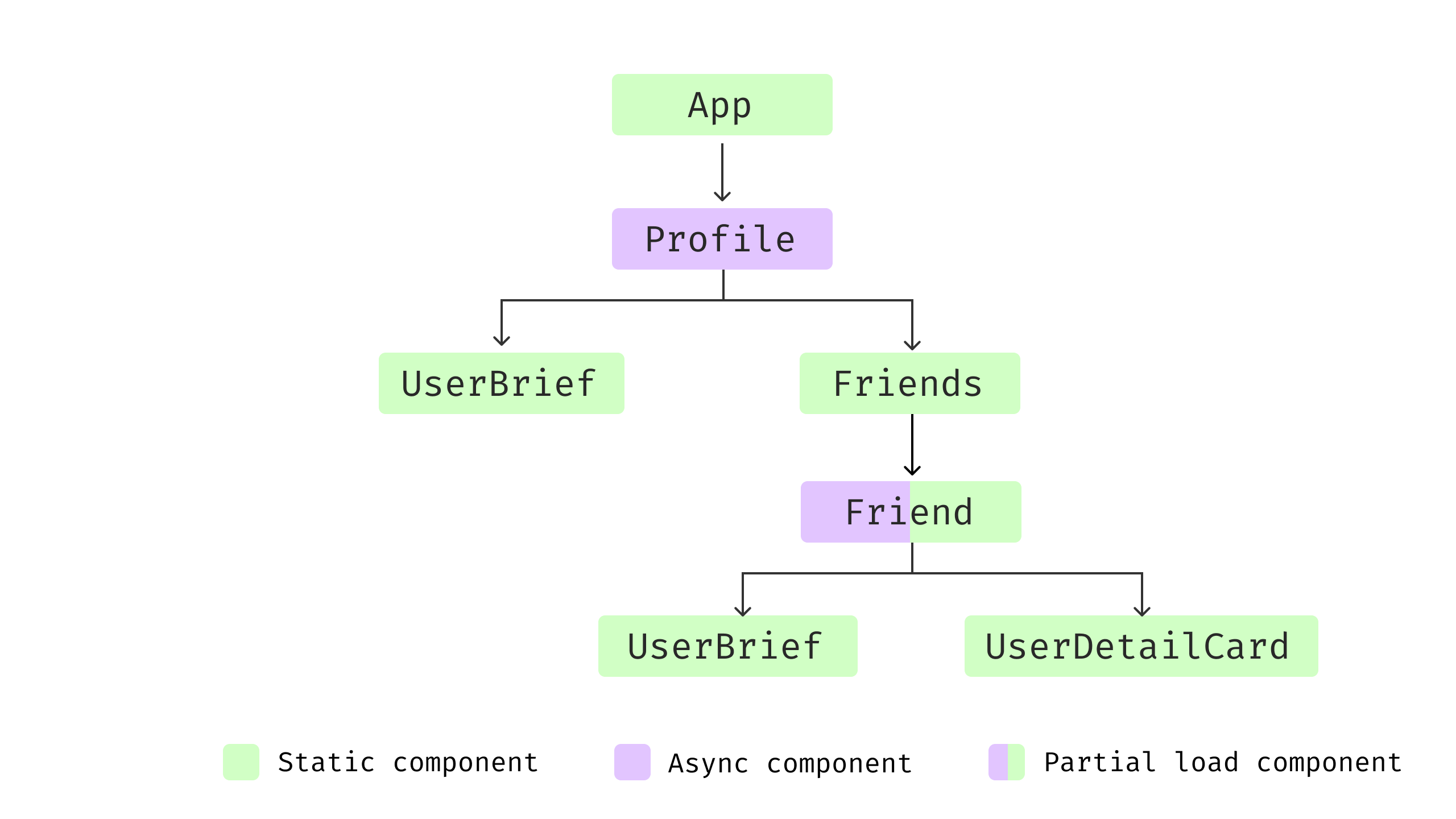
Determine 15: Element construction with
dynamic load
As the information fetching and loading is shifted to Pal
part, and for UserDetailCard
, it reads from the native
cache maintained by swr
.
import useSWR from "swr"; export perform UserDetailCard({ id }: { id: string }) { const { information: element, isLoading: loading } = useSWR( `/consumer/${id}/particulars`, () => getUserDetail(id) ); if (loading || !element) { returnLoading...
; } return ({/* render the consumer element*/}
); }
This part makes use of the useSWR
hook for information fetching,
making the UserDetailCard
dynamically load consumer particulars
based mostly on the given id
. useSWR
provides environment friendly
information fetching with caching, revalidation, and automated error dealing with.
The part shows a loading state till the information is fetched. As soon as
the information is out there, it proceeds to render the consumer particulars.
In abstract, we have already explored important information fetching methods:
Asynchronous State Handler , Parallel Information Fetching ,
Fallback Markup , Code Splitting and Prefetching . Elevating requests for parallel execution
enhances effectivity, although it is not at all times easy, particularly
when coping with elements developed by totally different groups with out full
visibility. Code splitting permits for the dynamic loading of
non-critical assets based mostly on consumer interplay, like clicks or hovers,
using prefetching to parallelize useful resource loading.
When to make use of it
Take into account making use of prefetching whenever you discover that the preliminary load time of
your software is changing into gradual, or there are numerous options that are not
instantly vital on the preliminary display screen however could possibly be wanted shortly after.
Prefetching is especially helpful for assets which can be triggered by consumer
interactions, comparable to mouse-overs or clicks. Whereas the browser is busy fetching
different assets, comparable to JavaScript bundles or belongings, prefetching can load
further information prematurely, thus getting ready for when the consumer really must
see the content material. By loading assets throughout idle occasions, prefetching makes use of the
community extra effectively, spreading the load over time relatively than inflicting spikes
in demand.
It’s sensible to comply with a basic guideline: do not implement complicated patterns like
prefetching till they’re clearly wanted. This could be the case if efficiency
points turn out to be obvious, particularly throughout preliminary masses, or if a big
portion of your customers entry the app from cell gadgets, which usually have
much less bandwidth and slower JavaScript engines. Additionally, take into account that there are different
efficiency optimization techniques comparable to caching at varied ranges, utilizing CDNs
for static belongings, and making certain belongings are compressed. These strategies can improve
efficiency with less complicated configurations and with out further coding. The
effectiveness of prefetching depends on precisely predicting consumer actions.
Incorrect assumptions can result in ineffective prefetching and even degrade the
consumer expertise by delaying the loading of really wanted assets.