Buyer assist calls maintain a wealth of data, however discovering the time to manually comb by these recordings for insights isn’t simple. Think about should you may immediately flip these lengthy recordings into clear summaries, monitor how the sentiment shifts all through the decision, and even get tailor-made insights based mostly on the way you wish to analyze the dialog. Sounds helpful?
On this article, we’ll stroll by making a sensible instrument I constructed SnapSynapse (click on right here), to do precisely that! Utilizing instruments like pyannote.audio for speaker diarization(identification), Whisper for transcription, and Gemini-1.5 Professional for producing AI-driven summaries, I’ll present how one can automate the method of turning assist name recordings into actionable insights. Alongside the best way, you’ll see methods to clear and refine transcriptions, generate customized summaries based mostly on person enter, and monitor sentiment developments—all with easy-to-follow code snippets. It is a hands-on information to constructing a instrument that goes past transcription that can assist you perceive and enhance your buyer assist expertise.
Studying Aims
- Perceive methods to use pyannote.audio for speaker diarization, separating totally different voices in buyer assist recordings.
- Be taught to generate correct transcriptions from audio information utilizing Whisper and clear them by eradicating filler phrases and irrelevant textual content.
- Uncover methods to create tailor-made summaries utilizing Gemini-1.5 Professional, with customizable prompts to suit totally different evaluation wants.
- Discover methods for performing sentiment evaluation on conversations and visualizing sentiment developments all through a name.
- Achieve hands-on expertise in constructing an automatic pipeline that processes audio information into structured insights, making it simpler to investigate and enhance buyer assist interactions.
This text was printed as part of the Knowledge Science Blogathon.
What’s SnapSynapse?
SnapSynapse is a helpful instrument for turning buyer assist calls into invaluable insights. It breaks down conversations by speaker, transcribes all the pieces, and highlights the general temper and key factors, so groups can rapidly perceive what clients want. Utilizing fashions like Pyannote for diarization, Whisper for transcription, and Gemini for summaries, SnapSynapse delivers clear summaries and sentiment developments with none trouble. It’s designed to assist assist groups join higher with clients and enhance service, one dialog at a time.
Key Options
Beneath are the necessary key options of SnapSynapse:
- Speaker diarization/identification
- Dialog transcript era
- Time Stamps era dialogue sensible
- Use case based mostly Abstract era
- Sentiment Evaluation scores
- Sentiment Evaluation by visualization
Constructing SnapSynapse: Core Options and Performance
On this part, we’ll discover the core options that make SnapSynapse a robust instrument for buyer assist evaluation. From mechanically diarizing and transcribing calls to producing dynamic dialog summaries, these options are constructed to boost assist crew effectivity. With its potential to detect sentiment developments and supply actionable insights, SnapSynapse simplifies the method of understanding buyer interactions.
In case, if you wish to try the entire supply code, confer with the information within the repo : repo_link
We’ll want OPEN AI API and GEMINI API to run this mission. You may get the API’s right here – Gemini API , OpenAI API
Venture circulation:
speaker diarization -> transcription -> time stamps -> cleansing -> summarization -> sentiment evaluation
Step1: Speaker Diarization and Transcription Technology
In step one, we’ll use a single script to take an audio file, separate the audio system (diarization), generate a transcription, and assign timestamps. Right here’s how the script works, together with a breakdown of the code and key features:
Overview of the Script
This Python script performs three primary duties in a single go:
- Speaker Diarization: Identifies totally different audio system in an audio file and separates their dialogue.
- Transcription: Converts every speaker’s separated audio segments into textual content.
- Timestamping: Provides timestamps for every spoken phase.
Imports and Setup
- We begin by importing essential libraries like pyannote.audio for speaker diarization, openai for transcription, and pydub to deal with audio segments.
- Setting variables are loaded utilizing dotenv, so we are able to securely retailer our OpenAI API key.
Most important Perform: Diarization + Transcription with Timestamps
The core perform, transcribe_with_diarization(), combines all of the steps:
- Diarization: Calls perform_diarization() to get speaker segments.
- Section Extraction: Makes use of pydub to chop the audio file into chunks based mostly on every phase’s begin and finish occasions.
- Transcription: For every chunk, it calls the Whisper mannequin through OpenAI’s API to get textual content transcriptions.
- Timestamp and Speaker Information: Every transcription is saved with its corresponding begin time, finish time, and speaker label.
def transcribe_with_diarization(file_path):
diarization_result = perform_diarization(file_path)
audio = AudioSegment.from_file(file_path)
transcriptions = []
for phase, _, speaker in diarization_result.itertracks(yield_label=True):
start_time_ms = int(phase.begin * 1000)
end_time_ms = int(phase.finish * 1000)
chunk = audio[start_time_ms:end_time_ms]
chunk_filename = f"{speaker}_segment_{int(phase.begin)}.wav"
chunk.export(chunk_filename, format="wav")
with open(chunk_filename, "rb") as audio_file:
transcription = consumer.audio.transcriptions.create(
mannequin="whisper-1",
file=audio_file,
response_format="json"
)
transcriptions.append({
"speaker": speaker,
"start_time": phase.begin,
"end_time": phase.finish,
"transcription": transcription.textual content
})
print(f"Transcription for {chunk_filename} by {speaker} accomplished.")
Saving the Output
- The ultimate transcriptions, together with speaker labels and timestamps, are saved to diarized_transcriptions.json, making a structured file of the dialog.
- Lastly, we run the perform on a take a look at audio file, test_audio_1.wav, to see the complete diarization and transcription course of in motion.
A Glimpse of the output generated and bought saved in diarized_transcription.py file:
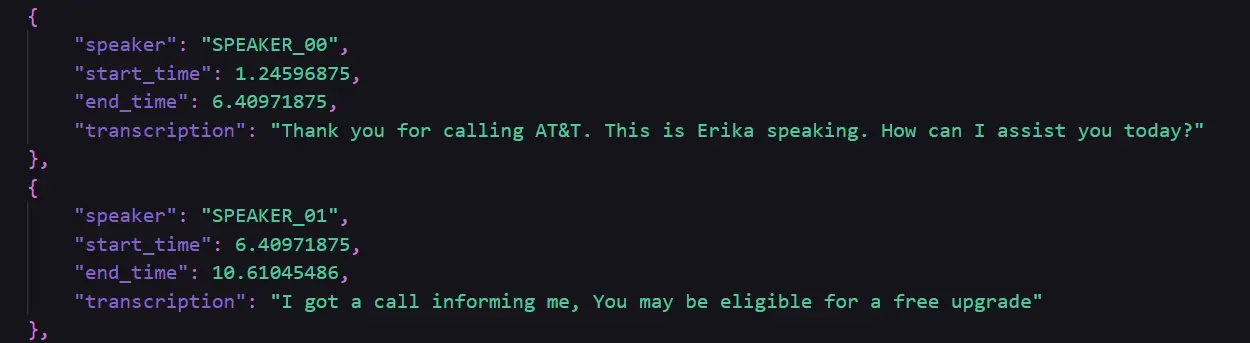
Step2: Cleansing of the Generated Transcription
- This file focuses on cleansing the transcriptions generated from the diarization and transcription course of.
- It hundreds the diarized transcriptions from a JSON file and removes widespread filler phrases like “um,” “uh,” and “you understand” to enhance readability.
- Moreover, it eliminates further white areas and normalizes the textual content to make the transcription extra concise and polished.
- After cleansing, the system saves the brand new transcriptions in a JSON file named cleaned_transcription.py, making certain that the information is prepared for additional evaluation or perception era.
# perform to wash the transcription textual content
def clean_transcription(textual content):
# Record of widespread filler phrases
filler_words = [
"um", "uh", "like", "you know", "actually", "basically", "I mean",
"sort of", "kind of", "right", "okay", "so", "well", "just"
]
# regex sample to match filler phrases (case insensitive)
filler_pattern = re.compile(r'b(' + '|'.be part of(filler_words) + r')b', re.IGNORECASE)
# Take away filler phrases
cleaned_text = filler_pattern.sub('', textual content)
# Take away further whitespace
cleaned_text = re.sub(r's+', ' ', cleaned_text).strip()
return cleaned_text
Step3: Producing Abstract utilizing GEMINI 1.5 professional
Within the subsequent step, we use the Gemini API to generate structured insights and summaries based mostly on the cleaned transcriptions. We make the most of the Gemini 1.5 professional mannequin for pure language processing to investigate buyer assist calls and supply actionable summaries.
Right here’s a breakdown of the performance:
- Mannequin Setup: The Gemini mannequin is configured utilizing the google.generativeai library, with the API key securely loaded. It helps producing insights based mostly on totally different immediate codecs.
- Prompts for Evaluation: A number of predefined prompts are designed to investigate numerous elements of the assist name, resembling common name summaries, speaker exchanges, complaints and resolutions, escalation wants, and technical concern troubleshooting.
- Generate Structured Content material: The perform generate_analysis() takes the cleaned transcription textual content and processes it utilizing one of many predefined prompts. It organizes the output into three sections: Abstract, Motion Gadgets, and Key phrases.
- Person Interplay: The script permits the person to select from a number of abstract codecs. The person’s selection determines which immediate is used to generate the insights from the transcription.
- Output Technology: After processing the transcription, the ensuing insights—organized right into a structured JSON format—are saved to a file. This structured information makes it simpler for assist groups to extract significant data from the decision.
A brief glimpse of various prompts used:
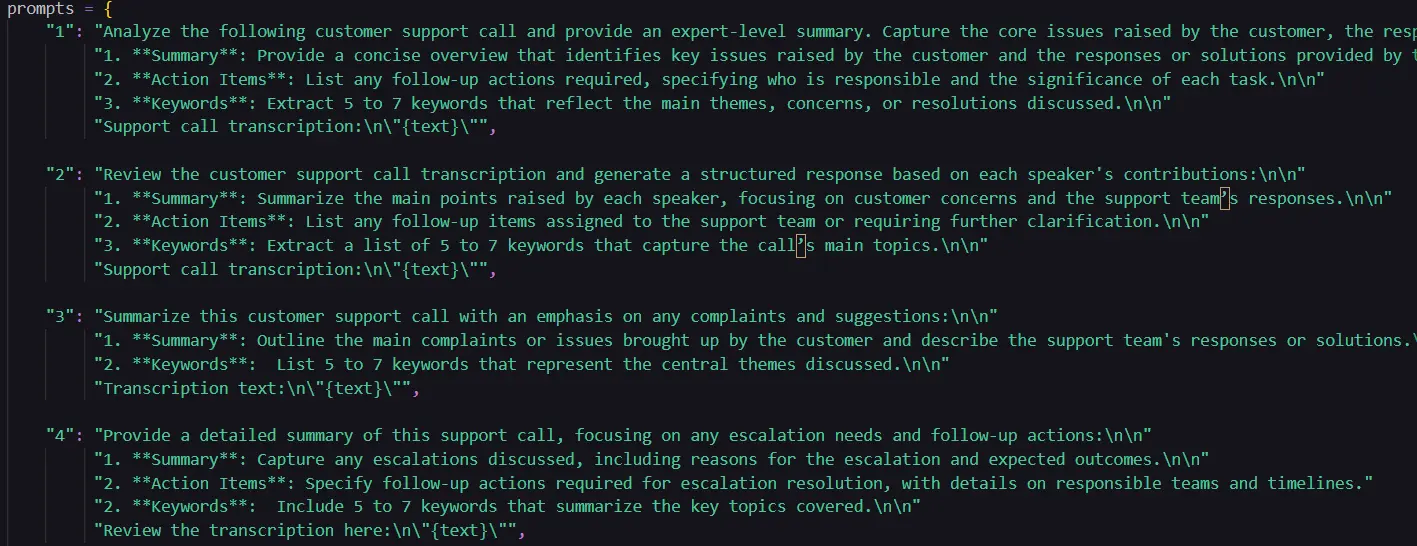
A glimpse of the output generated:
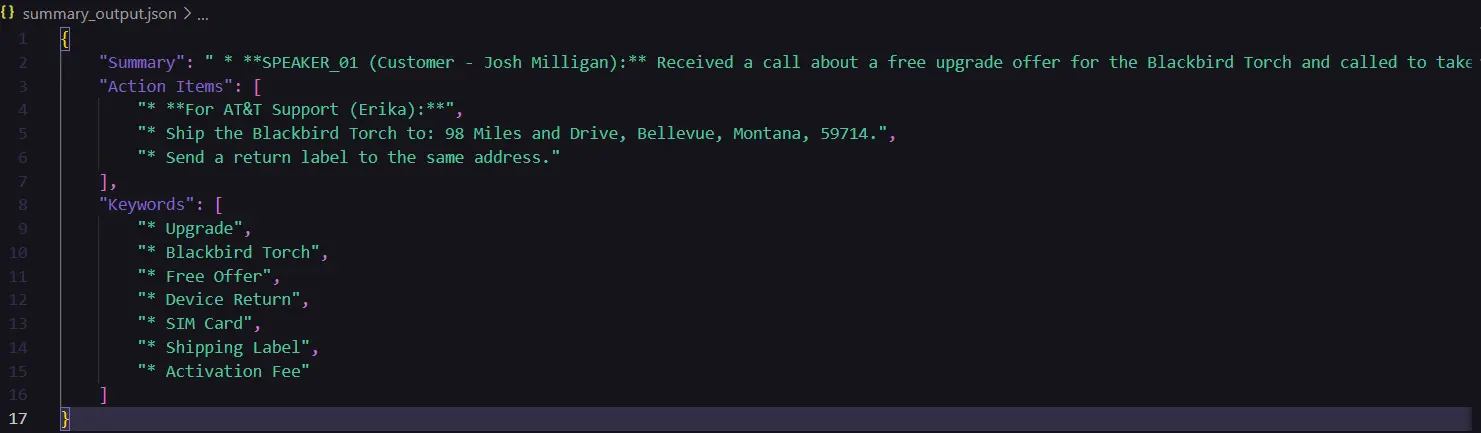
Step 4: Sentiment Evaluation
Additional, within the subsequent step we carry out sentiment evaluation on buyer assist name transcriptions to evaluate the emotional tone all through the dialog. It makes use of the VADER sentiment evaluation instrument from NLTK to find out sentiment scores for every phase of the dialog.
Right here’s a breakdown of the method:
- Sentiment Evaluation Utilizing VADER: The script makes use of SentimentIntensityAnalyzer from the VADER (Valence Conscious Dictionary and sEntiment Reasoner) lexicon. It assigns a sentiment rating for every phase, which features a compound rating indicating the general sentiment (optimistic, impartial, or adverse).
- Processing Transcription: The cleaned transcription is loaded from a JSON file. Every entry within the transcription is evaluated for sentiment, and the outcomes are saved with the speaker label and corresponding sentiment scores. The script calculates the entire sentiment rating, the common sentiment for the client and assist agent, and categorizes the general sentiment as Optimistic, Impartial, or Unfavourable.
- Sentiment Pattern Visualization: Utilizing Matplotlib, the script generates a line plot exhibiting the development of sentiment over time, with the x-axis representing the dialog segments and the y-axis exhibiting the sentiment rating.
- Output: The system saves the sentiment evaluation outcomes, together with the scores and total sentiment, to a JSON file for straightforward entry and evaluation later. It visualizes the sentiment development in a plot to offer an summary of the emotional dynamics through the assist name.
Code used for calculating the general sentiment rating
# Calculate the general sentiment rating
overall_sentiment_score = total_compound / len(sentiment_results)
# Calculate common sentiment for Buyer and Agent
average_customer_sentiment = customer_sentiment / customer_count if customer_count else 0
average_agent_sentiment = agent_sentiment / agent_count if agent_count else 0
# Decide the general sentiment as optimistic, impartial, or adverse
if overall_sentiment_score > 0.05:
overall_sentiment = "Optimistic"
elif overall_sentiment_score < -0.05:
overall_sentiment = "Unfavourable"
else:
overall_sentiment = "Impartial"
Code used for producing the plot
def plot_sentiment_trend(sentiment_results):
# Extract compound sentiment scores for plotting
compound_scores = [entry['sentiment']['compound'] for entry in sentiment_results]
# Create a single line plot exhibiting sentiment development
plt.determine(figsize=(12, 6))
plt.plot(compound_scores, coloration="purple", linestyle="-", marker="o", markersize=5, label="Sentiment Pattern")
plt.axhline(0, coloration="gray", linestyle="--") # Add a zero line for impartial sentiment
plt.title("Sentiment Pattern Over the Buyer Help Dialog", fontsize=16, fontweight="daring", coloration="darkblue")
plt.xlabel("Section Index")
plt.ylabel("Compound Sentiment Rating")
plt.grid(True, linestyle="--", alpha=0.5)
plt.legend()
plt.present()
Sentiment Evaluation Scores generated:

Sentiment Evaluation Plot generated:
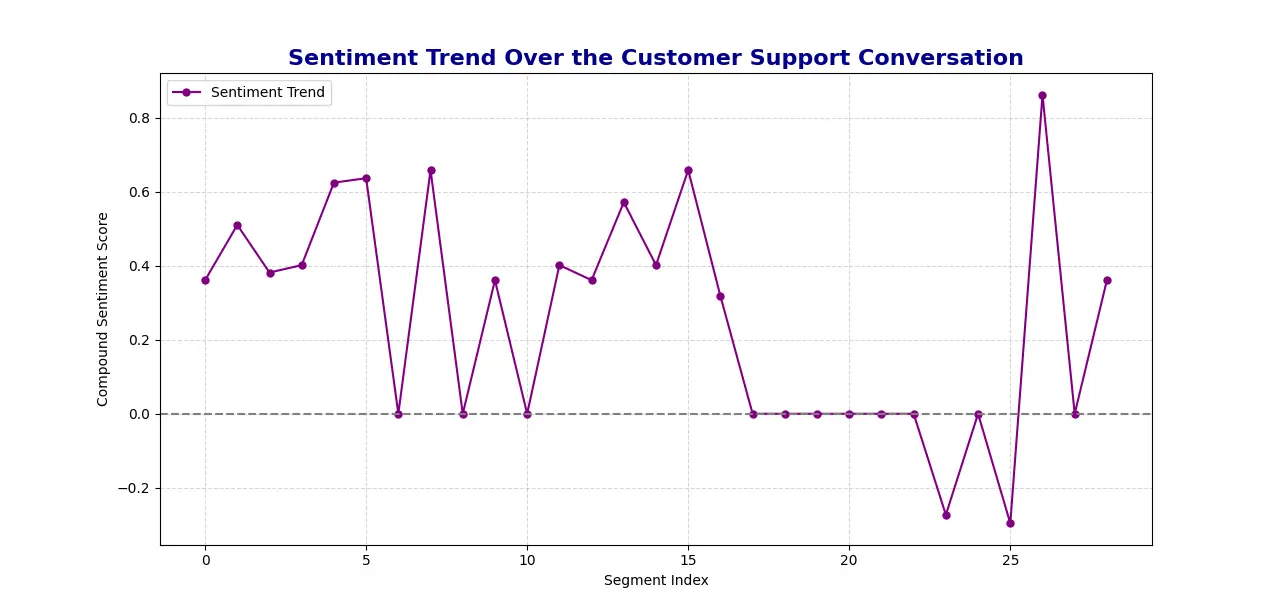
Setting Up SnapSynapse: A Step-by-Step Information
You’ll find the code repository right here – repo_link
Now, let’s stroll by methods to arrange and run SnapSynapse in your native machine:
Step1: Clone the Repository
Begin by cloning the mission repository to your native machine to start utilizing SnapSynapse. This supplies entry to the applying’s supply code and all its important parts.
git clone https://github.com/Keerthanareddy95/SnapSynapse.git
cd SnapSynapse
Step2: Setup the Digital Setting
A digital atmosphere helps isolate dependencies and ensures your mission runs easily. This step units up an unbiased workspace for SnapSynapse to function with out interference from different packages.
# For Home windows:
python -m venv venv
# For macOS and Linux:
python3 -m venv venv
Step3: Activate the Digital Setting
# For Home windows:
.venvScriptsactivate
# For macOS and Linux:
supply venv/bin/activate
Step4: Set up Required Dependencies
With the digital atmosphere in place, the following step is to put in all essential libraries and instruments. These dependencies allow the core functionalities of SnapSynapse, together with transcript era, speaker diarization, time stamp era, abstract era, sentiment evaluation scores, visualization and extra.
pip set up -r necessities.txt
Step5: Arrange the Setting Variables
To leverage AI-driven insights, diarization, transcription and summarization and also you’ll have to configure API keys for Google Gemini and OPEN AI Whisper.
Create a .env file within the root of the mission and add your API keys for Google Gemini and OPEN AI Whisper.
GOOGLE_API_KEY="your_google_api_key"
OPENAI_API_KEY="your_open_ai_api_key"
Step6: Run the Software
- Begin by working the transcription.py file: This file performs the transcription era, speaker diarization and time stamp era. And it saves the output in a json file named diarized_transcriptions.json.
- Subsequent, run the cleansing.py file: This file will take the diarized_transcriptions.py file as enter and cleans the transcription and saves the ends in cleaned_transcription.json file.
- Additional, run the abstract.py file: right here you could point out the GEMINI API key. This file will take the cleaned_transcription.py file as enter and prompts the person to enter the fashion of abstract they wish to generate based mostly on their use case. Primarily based on the person enter, the system passes the corresponding immediate to GEMINI, which generates the abstract. The system then shops the generated abstract in a JSON file named
summary_output.json
. - Lastly, run the sentiment_analysis.py file: Working this file will generate the general sentiment scores and likewise a graphical illustration of the sentiment evaluation scores and the way they progressed by the audio file.
Allow us to now look onto the instruments utilized in growth for SnapSynapse beneath:
- pyannote.audio : Gives the Pipeline module for performing speaker diarization, which separates totally different audio system in an audio file.
- openai: Used to work together with OpenAI’s API for transcription through the Whisper mannequin.
- pydub (AudioSegment): Processes audio information, permitting segmentation and export of audio chunks by speaker.
- google.generativeai: A library to entry Google Gemini fashions, used right here to generate structured summaries and insights from buyer assist transcriptions.
- NLTK (Pure Language Toolkit): A library for pure language processing, particularly used right here to import the SentimentIntensityAnalyzer from VADER to investigate sentiment within the audio file.
- Matplotlib: A visualization library usually used with plt, included right here for visualization of the sentiment all through the audio file.
Conclusion
In a nutshell, SnapSynapse revolutionizes buyer assist evaluation by remodeling uncooked name recordings into actionable insights. From speaker diarization and transcription to producing a structured abstract and sentiment evaluation, SnapSynapse streamlines each step to ship a complete view of buyer interactions. With the ability of the Gemini mannequin’s tailor-made prompts and detailed sentiment monitoring, customers can simply receive summaries and developments that spotlight key insights and assist outcomes.
An enormous shoutout to Google Gemini, Pyannote Audio, and Whisper for powering this mission with their modern instruments!
You possibly can try the repo right here.
Key Takeaways
- SnapSynapse permits customers to course of buyer assist calls end-to-end—from diarizing and transcribing to producing summaries.
- With 5 distinct immediate decisions, customers can tailor summaries to particular wants, whether or not specializing in points, motion gadgets, or technical assist. This function helps learners discover immediate engineering and experiment with how totally different inputs affect AI-generated outputs.
- SnapSynapse tracks sentiment developments all through conversations, offering a visible illustration of tone shifts that assist customers higher perceive buyer satisfaction. For learners, it’s an opportunity to use NLP methods and discover ways to interpret sentiment information in real-world purposes.
- SnapSynapse automates transcription cleanup and evaluation, making buyer assist insights simply accessible for quicker, data-driven choices. Learners profit from seeing how automation can streamline information processing, permitting them to concentrate on superior insights somewhat than repetitive duties.
Regularly Requested Questions
A. SnapSynapse can deal with audio information of the codecs mp3 and wav.
A. SnapSynapse makes use of Whisper for transcription, adopted by a cleanup course of that removes filler phrases, pauses, and irrelevant content material.
A. Sure! SnapSynapse gives 5 distinct immediate choices, permitting you to decide on a abstract format tailor-made to your wants. These embody focus areas like motion gadgets, escalation wants, and technical points.
A. SnapSynapse’s sentiment evaluation assesses the emotional tone of the dialog, offering a sentiment rating and a development graph.
A. Buyer Name Evaluation makes use of AI-powered instruments to transcribe, analyze, and extract invaluable insights from buyer interactions, serving to companies enhance service, determine developments, and improve buyer satisfaction.
A. By buyer name evaluation, companies can acquire a deeper understanding of buyer sentiment, widespread points, and agent efficiency, resulting in extra knowledgeable choices and improved customer support methods.
The media proven on this article will not be owned by Analytics Vidhya and is used on the Creator’s discretion.